Define Auth challenge Lambda trigger
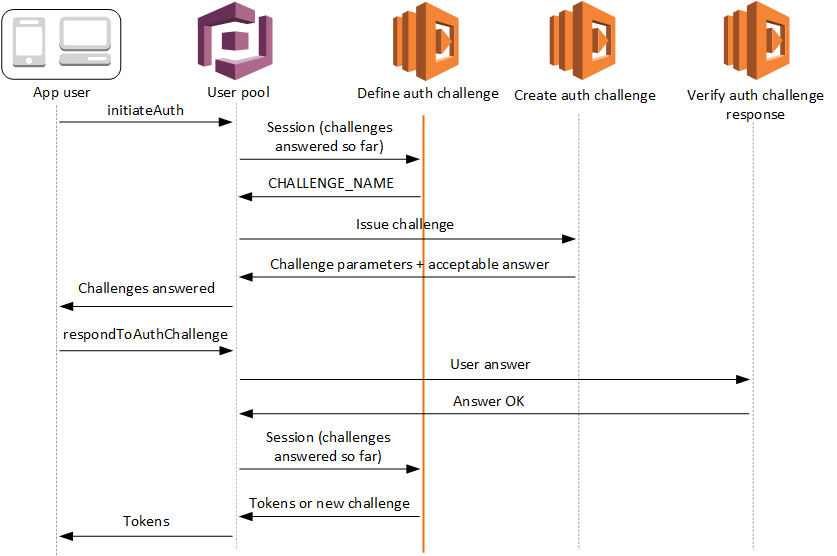
- Define auth challenge
-
Amazon Cognito invokes this trigger to initiate the custom authentication flow.
The request for this Lambda trigger contains session
. The session
parameter is an array that contains all of the challenges that are presented to the user in
the current authentication process. The request also includes the corresponding result. The
session
array stores challenge details (ChallengeResult
) in
chronological order. The challenge session[0]
represents the first challenge
that the user receives.
You can have Amazon Cognito verify user passwords before it issues your custom challenges. Any Lambda triggers associated in the Authentication category of request-rate quotas will run when you perform SRP authentication in a custom challenge flow. Here is an overview of the process:
-
Your app initiates sign-in by calling
InitiateAuth
orAdminInitiateAuth
with theAuthParameters
map. Parameters must includeCHALLENGE_NAME: SRP_A,
and values forSRP_A
andUSERNAME
. -
Amazon Cognito invokes your define auth challenge Lambda trigger with an initial session that contains
challengeName: SRP_A
andchallengeResult: true
. -
After receiving those inputs, your Lambda function responds with
challengeName: PASSWORD_VERIFIER
,issueTokens: false
,failAuthentication: false
. -
If the password verification succeeds, Amazon Cognito invokes your Lambda function again with a new session containing
challengeName: PASSWORD_VERIFIER
andchallengeResult: true
. -
To initiate your custom challenges, your Lambda function responds with
challengeName: CUSTOM_CHALLENGE
,issueTokens: false
, andfailAuthentication: false
. If you don't want to start your custom auth flow with password verification, you can initiate sign-in with theAuthParameters
map includingCHALLENGE_NAME: CUSTOM_CHALLENGE
. -
The challenge loop repeats until all challenges are answered.
Define Auth challenge Lambda trigger parameters
The request that Amazon Cognito passes to this Lambda function is a combination of the parameters below and the common parameters that Amazon Cognito adds to all requests.
Define Auth challenge request parameters
When Amazon Cognito invokes your Lambda function, Amazon Cognito provides the following parameters:
- userAttributes
-
One or more name-value pairs that represent user attributes.
- userNotFound
-
A Boolean that Amazon Cognito populates when
PreventUserExistenceErrors
is set toENABLED
for your user pool client. A value oftrue
means that the user id (username, email address, and other details) did not match any existing users. WhenPreventUserExistenceErrors
is set toENABLED
, the service doesn't inform the app of nonexistent users. We recommend that your Lambda functions maintain the same user experience and account for latency. This way, the caller can't detect different behavior when the user exists or doesn’t exist. - session
-
An array of
ChallengeResult
elements. Each contains the following elements:- challengeName
-
One of the following challenge types:
CUSTOM_CHALLENGE
,SRP_A
,PASSWORD_VERIFIER
,SMS_MFA, DEVICE_SRP_AUTH
,DEVICE_PASSWORD_VERIFIER
, orADMIN_NO_SRP_AUTH
.When your define auth challenge function issues a
PASSWORD_VERIFIER
challenge for a user who has set up multifactor authentication, Amazon Cognito follows it up with anSMS_MFA
challenge. In your function, include handling for input events fromSMS_MFA
challenges. You don't need to invoke theSMS_MFA
challenge from your define auth challenge function.Important
When your function is determining whether a user has successfully authenticated and you should issue them tokens, always check
challengeName
in your define auth challenge function and verify that it matches the expected value. - challengeResult
-
Set to
true
if the user successfully completed the challenge, orfalse
otherwise. - challengeMetadata
-
Your name for the custom challenge. Used only if
challengeName
isCUSTOM_CHALLENGE
.
- clientMetadata
-
One or more key-value pairs that you can provide as custom input to the Lambda function that you specify for the define auth challenge trigger. To pass this data to your Lambda function, you can use the
ClientMetadata
parameter in the AdminRespondToAuthChallenge and RespondToAuthChallenge API operations. The request that invokes the define auth challenge function doesn't include data passed in the ClientMetadata parameter in AdminInitiateAuth and InitiateAuth API operations.
Define Auth challenge response parameters
In the response, you can return the next stage of the authentication process.
- challengeName
-
A string that contains the name of the next challenge. If you want to present a new challenge to your user, specify the challenge name here.
- issueTokens
-
If you determine that the user has completed the authentication challenges sufficiently, set to
true
. If the user has not met the challenges sufficiently, set tofalse
. - failAuthentication
-
If you want to end the current authentication process, set to
true
. To continue the current authentication process, set tofalse
.
Define Auth challenge example
This example defines a series of challenges for authentication and issues tokens only if the user has completed all of the challenges successfully.