Post authentication Lambda trigger
Because Amazon Cognito invokes this trigger after signing in a user, you can add custom logic after Amazon Cognito authenticates the user.
Topics
Authentication flow overview
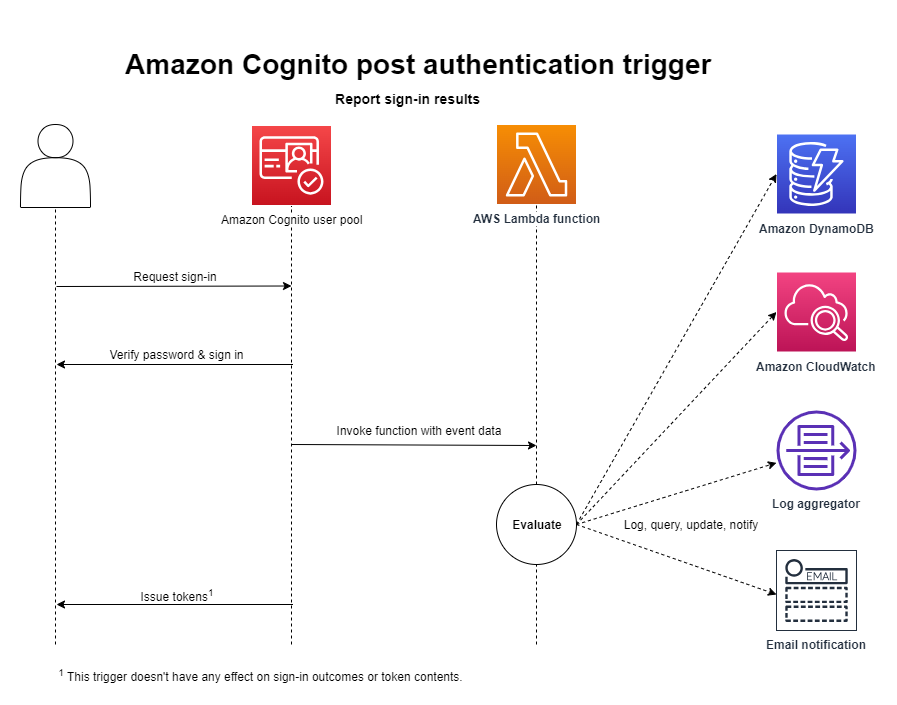
For more information, see User pool authentication flow.
Post authentication Lambda trigger parameters
The request that Amazon Cognito passes to this Lambda function is a combination of the parameters below and the common parameters that Amazon Cognito adds to all requests.
Post authentication request parameters
- newDeviceUsed
-
This flag indicates if the user has signed in on a new device. Amazon Cognito only sets this flag if the remembered devices value of the user pool is
Always
orUser Opt-In
. - userAttributes
-
One or more name-value pairs representing user attributes.
- clientMetadata
-
One or more key-value pairs that you can provide as custom input to the Lambda function that you specify for the post authentication trigger. To pass this data to your Lambda function, you can use the ClientMetadata parameter in the AdminRespondToAuthChallenge and RespondToAuthChallenge API actions. Amazon Cognito doesn't include data from the ClientMetadata parameter in AdminInitiateAuth and InitiateAuth API operations in the request that it passes to the post authentication function.
Post authentication response parameters
Amazon Cognito doesn't expect any additional return information in the response. Your function can use API operations to query and modify your resources, or record event metadata to an external system.
Authentication tutorials
Immediately after Amazon Cognito signs in a user, it activates the post authentication Lambda function. See these sign-in tutorials for JavaScript, Android, and iOS.
Platform | Tutorial |
---|---|
JavaScript Identity SDK | Sign in users with JavaScript |
Android Identity SDK | Sign in users with Android |
iOS Identity SDK | Sign in users with iOS |
Post authentication example
This post authentication sample Lambda function sends data from a successful sign-in to CloudWatch Logs.
Amazon Cognito passes event information to your Lambda function. The function then returns the same event object to Amazon Cognito, with any changes in the response. In the Lambda console, you can set up a test event with data that is relevant to your Lambda trigger. The following is a test event for this code sample: