Pre sign-up Lambda trigger
Shortly before Amazon Cognito signs up a new user, it activates the pre sign-up AWS Lambda function. As part of the sign-up process, you can use this function to perform custom validation and, based on the results of your validation, accept or deny the registration request.
Topics
Pre sign-up Lambda flows
Client sign-up flow
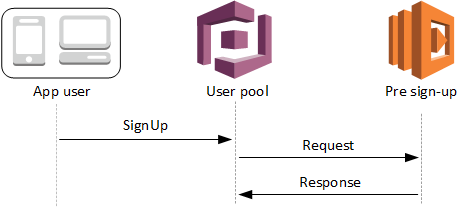
Server sign-up flow

The request includes validation data from the client. This data comes from the
ValidationData
values passed to the user pool SignUp and
AdminCreateUser API methods.
Pre sign-up Lambda trigger parameters
The request that Amazon Cognito passes to this Lambda function is a combination of the parameters below and the common parameters that Amazon Cognito adds to all requests.
Pre sign-up request parameters
- userAttributes
-
One or more name-value pairs representing user attributes. The attribute names are the keys.
- validationData
-
One or more key-value pairs with user attribute data that your app passed to Amazon Cognito in the request to create a new user. Send this information to your Lambda function in the ValidationData parameter of your AdminCreateUser or SignUp API request.
Amazon Cognito doesn't set your ValidationData data as attributes of the user that you create. ValidationData is temporary user information that you supply for the purposes of your pre sign-up Lambda trigger.
- clientMetadata
-
One or more key-value pairs that you can provide as custom input to the Lambda function that you specify for the pre sign-up trigger. You can pass this data to your Lambda function by using the ClientMetadata parameter in the following API actions: AdminCreateUser, AdminRespondToAuthChallenge, ForgotPassword, and SignUp.
Pre sign-up response parameters
In the response, you can set autoConfirmUser
to true
if
you want to auto-confirm the user. You can set autoVerifyEmail
to
true
to auto-verify the user's email. You can set
autoVerifyPhone
to true
to auto-verify the user's
phone number.
Note
Response parameters autoVerifyPhone
, autoVerifyEmail
and autoConfirmUser
are ignored by Amazon Cognito when the pre sign-up Lambda
function is triggered by the AdminCreateUser
API.
- autoConfirmUser
-
Set to
true
to auto-confirm the user, orfalse
otherwise. - autoVerifyEmail
-
Set to
true
to set as verified the email address of a user who is signing up, orfalse
otherwise. IfautoVerifyEmail
is set totrue
, theemail
attribute must have a valid, non-null value. Otherwise an error will occur and the user will not be able to complete sign-up.If the
email
attribute is selected as an alias, an alias will be created for the user's email address whenautoVerifyEmail
is set. If an alias with that email address already exists, the alias will be moved to the new user and the previous user's email address will be marked as unverified. For more information, see Customizing sign-in attributes. - autoVerifyPhone
-
Set to
true
to set as verified the phone number of a user who is signing up, orfalse
otherwise. IfautoVerifyPhone
is set totrue
, thephone_number
attribute must have a valid, non-null value. Otherwise an error will occur and the user will not be able to complete sign-up.If the
phone_number
attribute is selected as an alias, an alias will be created for the user's phone number whenautoVerifyPhone
is set. If an alias with that phone number already exists, the alias will be moved to the new user and the previous user's phone number will be marked as unverified. For more information, see Customizing sign-in attributes.
Sign-up tutorials
The pre sign-up Lambda function is triggered before user sign-up. See these Amazon Cognito sign-up tutorials for JavaScript, Android, and iOS.
Platform | Tutorial |
---|---|
JavaScript Identity SDK | Sign up users with JavaScript |
Android Identity SDK | Sign up users with Android |
iOS Identity SDK | Sign up users with iOS |
Pre sign-up example: Auto-confirm users from a registered domain
You can use the pre sign-up Lambda trigger to add custom logic that validates new users who sign up for your user pool. This is a sample JavaScript program that shows how to sign up a new user. It invokes a pre sign-up Lambda trigger as part of the authentication.
This is a sample Lambda trigger called just before sign-up with the user pool pre sign-up Lambda trigger. It uses a custom attribute custom:domain to automatically confirm new users from a particular email domain. Any new users not in the custom domain will be added to the user pool, but not automatically confirmed.
Amazon Cognito passes event information to your Lambda function. The function then returns the same event object to Amazon Cognito, with any changes in the response. In the Lambda console, you can set up a test event with data that is relevant to your Lambda trigger. The following is a test event for this code sample:
Pre sign-up example: Auto-confirm and auto-verify all users
This example confirms all users and sets the user's email
and
phone_number
attributes to verified if the attribute is present. Also,
if aliasing is enabled, aliases will be created for phone_number
and
email
when auto-verify is set.
Note
If an alias with the same phone number already exists, the alias will be moved to
the new user, and the previous user's phone_number
will be marked as
unverified. The same is true for email addresses. To prevent this from happening,
you can use the user pools ListUsers API to see if there is an existing user who is already using
the new user's phone number or email address as an alias.
Amazon Cognito passes event information to your Lambda function. The function then returns the same event object to Amazon Cognito, with any changes in the response. In the Lambda console, you can set up a test event with data that is relevant to your Lambda trigger. The following is a test event for this code sample:
Pre sign-up example: Deny sign-up if user name has fewer than five characters
This example checks the length of the user name in a sign-up request. The example returns an error if the user has entered a name less than five characters long.
Amazon Cognito passes event information to your Lambda function. The function then returns the same event object to Amazon Cognito, with any changes in the response. In the Lambda console, you can set up a test event with data that is relevant to your Lambda trigger. The following is a test event for this code sample: