Optimizing static initialization
Static initialization happens before the handler code starts running in a function. This is the “INIT” code that happens outside of the handler. This code is often used to import libraries and dependencies, set up configuration and initialize connections to other services.
The following Python example shows importing, and configuring modules, and creating the S3 client during the "INIT" phase, before the lambda-handler function runs during invoke.
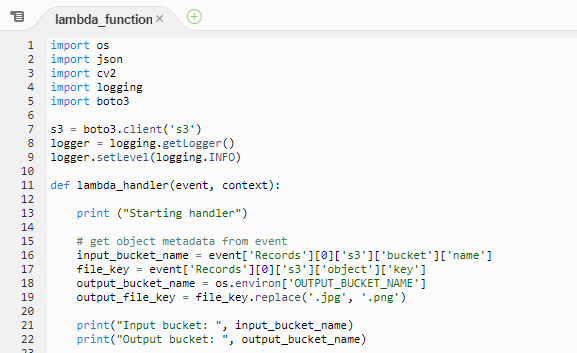
In our analyses of Lambda performance across production invocations, data shows that the largest contributor of latency before function execution comes from INIT code. The section that developers can control the most can also have the biggest impact on the duration of a cold start.
The INIT code runs when a new execution environment is run for the first time, and also whenever a function scales up and the Lambda service is creating new environments for the function. The initialization code is not run again if an invocation uses a warm execution environment. This portion of the cold start is influenced by:
-
The size of the function package, in terms of imported libraries and dependencies, and Lambda layers.
-
The amount of code and initialization work.
-
The performance of libraries and other services in setting up connections and other resources.
There are a number of steps that developers can take to optimize this portion of a cold start. If a function has many objects and connections, you may be able to rearchitect a single function into multiple, specialized functions. These are individually smaller and have less INIT code.
It’s important that functions only import the libraries and dependencies that they need. For example, if you only use Amazon DynamoDB in the AWS SDK, you can require an individual service instead of the entire SDK. Compare the following three examples:
// Instead of const AWS = require('aws-sdk'), use: const DynamoDB = require('aws-sdk/clients/dynamodb') // Instead of const AWSXRay = require('aws-xray-sdk'), use: const AWSXRay = require('aws-xray-sdk-core') // Instead of const AWS = AWSXRay.captureAWS(require('aws-sdk')), use: const dynamodb = new DynamoDB.DocumentClient() AWSXRay.captureAWSClient(dynamodb.service)
In testing, importing the DynamoDB library instead of the entire AWS SDK was 125 ms faster. Importing the X-Ray core library was 5 ms faster than the X-Ray SDK. Similarly, when wrapping a service initialization, preparing a DocumentClient before wrapping showed a 140-ms gain. Version 3 of the AWS SDK for JavaScript
Static initialization is often the best place to open database connections to allow a function to reuse connections over multiple invocations to the same execution environment. However, you may have large numbers of objects that are only used in certain execution paths in your function. In this case, you can lazily load variables in the global scope to reduce the static initialization duration.
Global variables should be avoided for context-specific information per invocation. If your function has a global variable that is used only for the lifetime of a single invocation and is reset for the next invocation, use a variable scope that is local to the handler. Not only does this prevent global variable leaks across invocations, it also improves the static initialization performance.