REST API에 대한 데이터 모델
API Gateway에서 모델은 페이로드의 데이터 구조를 정의합니다. API Gateway에서 모델은 JSON 스키마 드래프트 4
{ "id": 1, "type": "dog", "price": 249.99 }
데이터에는 반려동물의 id
, type
및 price
가 포함되어 있습니다. 이 데이터의 모델을 통해 다음을 수행할 수 있습니다.
기본 요청 확인을 사용합니다.
데이터 변환을 위한 매핑 템플릿을 생성합니다.
SDK를 생성할 때 사용자 정의 데이터 유형(UDT)을 생성합니다.
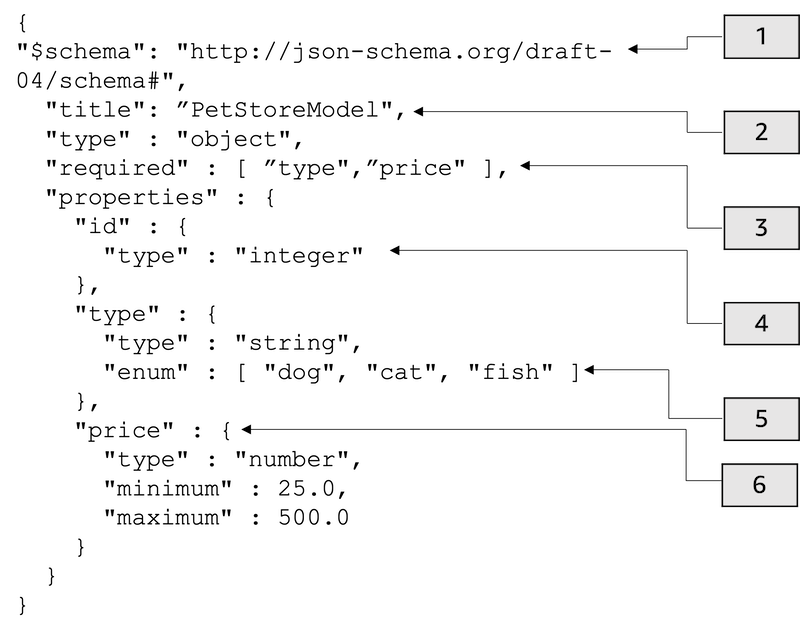
이 모델의 경우:
-
$schema
객체는 유효한 JSON 스키마 버전 식별자를 나타냅니다. 이 스키마는 JSON 스키마 드래프트 v4입니다. -
title
객체는 사람이 읽을 수 있는 모델 식별자입니다. 이 제목은PetStoreModel
입니다. -
기본 요청 확인에서는
required
확인 키워드에type
및price
가 필요합니다. -
모델의
properties
은id
,type
,price
입니다. 각 객체에는 모델에 설명된 속성이 있습니다. -
type
객체에는dog
,cat
, 또는fish
값만 있을 수 있습니다. -
price
객체는 숫자이며minimum
25,maximum
500으로 제한됩니다.
1 { 2 "$schema": "http://json-schema.org/draft-04/schema#", 3 "title": "PetStoreModel", 4 "type" : "object", 5 "required" : [ "price", "type" ], 6 "properties" : { 7 "id" : { 8 "type" : "integer" 9 }, 10 "type" : { 11 "type" : "string", 12 "enum" : [ "dog", "cat", "fish" ] 13 }, 14 "price" : { 15 "type" : "number", 16 "minimum" : 25.0, 17 "maximum" : 500.0 18 } 19 } 20 }
이 모델의 경우:
-
2번째 줄에서
$schema
객체는 유효한 JSON 스키마 버전 식별자를 나타냅니다. 이 스키마는 JSON 스키마 드래프트 v4입니다. -
3번째 줄에서
title
객체는 사람이 읽을 수 있는 모델 식별자입니다. 이 제목은PetStoreModel
입니다. -
5번째 줄에서 기본 요청 확인에서는
required
확인 키워드에type
및price
가 필요합니다. -
6~17번째 줄에서 모델의
properties
은id
,type
,price
입니다. 각 객체에는 모델에 설명된 속성이 있습니다. -
12번째 줄에서
type
객체에는dog
,cat
또는fish
값만 있을 수 있습니다. -
14~17번째 줄에서
price
객체는 숫자이며minimum
25,maximum
500으로 제한됩니다.
더 복잡한 모델 생성
$ref
프리미티브를 사용하여 더 긴 모델에 대해 재사용 가능한 정의를 만들 수 있습니다. 예를 들어, price
객체를 설명하는 definitions
섹션에서 Price
라는 정의를 생성할 수 있습니다. $ref
의 값은 Price
정의입니다.
{ "$schema" : "http://json-schema.org/draft-04/schema#", "title" : "PetStoreModelReUsableRef", "required" : ["price", "type" ], "type" : "object", "properties" : { "id" : { "type" : "integer" }, "type" : { "type" : "string", "enum" : [ "dog", "cat", "fish" ] }, "price" : { "$ref": "#/definitions/Price" } }, "definitions" : { "Price": { "type" : "number", "minimum" : 25.0, "maximum" : 500.0 } } }
외부 모델 파일에 정의된 다른 모델 스키마를 참조할 수도 있습니다. $ref
속성 값을 모델의 위치로 설정합니다. 다음 예시에서는 Price
모델이 a1234
API의 PetStorePrice
모델에 정의되어 있습니다.
{ "$schema" : "http://json-schema.org/draft-04/schema#", "title" : "PetStorePrice", "type": "number", "minimum": 25, "maximum": 500 }
더 긴 모델은 PetStorePrice
모델을 참조할 수 있습니다.
{ "$schema" : "http://json-schema.org/draft-04/schema#", "title" : "PetStoreModelReusableRefAPI", "required" : [ "price", "type" ], "type" : "object", "properties" : { "id" : { "type" : "integer" }, "type" : { "type" : "string", "enum" : [ "dog", "cat", "fish" ] }, "price" : { "$ref": "https://apigateway.amazonaws.com/restapis/a1234/models/PetStorePrice" } } }
출력 데이터 모델 사용
데이터를 변환하면 통합 응답에서 페이로드 모델을 정의할 수 있습니다. 페이로드 모델은 SDK를 생성할 때 사용할 수 있습니다. Java, Objective-C 또는 Swift 등 강력한 형식의 언어에서 객체는 사용자 정의 데이터 형식(UDT)에 해당합니다. SDK를 생성할 때 데이터 모델과 함께 제공하면 API Gateway가 이러한 UDT를 생성합니다. 데이터 변환에 대한 자세한 내용은 REST API용 매핑 템플릿 섹션을 참조하세요.
다음 예시는 통합 응답의 출력 데이터입니다.
{ [ { "description" : "Item 1 is a dog.", "askingPrice" : 249.99 }, { "description" : "Item 2 is a cat.", "askingPrice" : 124.99 }, { "description" : "Item 3 is a fish.", "askingPrice" : 0.99 } ] }
다음 예시는 출력 데이터를 설명하는 페이로드 모델입니다.
{ "$schema": "http://json-schema.org/draft-04/schema#", "title": ”PetStoreOutputModel", "type" : "object", "required" : [ "description", "askingPrice" ], "properties" : { "description" : { "type" : "string" }, "askingPrice" : { "type" : "number", "minimum" : 25.0, "maximum" : 500.0 } } }
이 모델에서는 PetStoreOutputModel[i].description
및 PetStoreOutputModel[i].askingPrice
속성을 읽음으로써 description
및 askingPrice
속성 값을 검색하도록 SDK를 호출할 수 있습니다. 모델이 제공되지 않는 경우, API Gateway는 빈 모델을 사용하여 기본 UDT를 생성합니다.
다음 단계
-
이 섹션에서는 이 주제에 제시된 개념에 대해 자세히 알아보는 데 사용할 수 있는 리소스를 제공합니다.
요청 확인 자습서를 따라 수행할 수 있습니다.
-
데이터 변환 및 매핑 템플릿(REST API용 매핑 템플릿)에 대한 자세한 정보를 얻을 수 있습니다.
-
더 복잡한 데이터 모델도 확인할 수 있습니다. API Gateway용 데이터 모델 및 매핑 템플릿 예시 섹션을 참조하세요.