Detecting text in an image
You can provide an input image as an image byte array (base64-encoded image bytes), or as an Amazon S3 object. In this procedure, you upload a JPEG or PNG image to your S3 bucket and specify the file name.
To detect text in an image (API)
-
If you haven't already, complete the following prerequisites.
-
Create or update a user with
AmazonRekognitionFullAccess
andAmazonS3ReadOnlyAccess
permissions. For more information, see Step 1: Set up an AWS account and create a User. -
Install and configure the AWS Command Line Interface and the AWS SDKs. For more information, see Step 2: Set up the AWS CLI and AWS SDKs.
-
-
Upload the image that contains text to your S3 bucket.
For instructions, see Uploading Objects into Amazon S3 in the Amazon Simple Storage Service User Guide.
-
Use the following examples to call the
DetectText
operation.
DetectText operation request
In the DetectText
operation, you supply an input image either as a
base64-encoded byte array or as an image stored in an Amazon S3 bucket. The following
example JSON request shows the image loaded from an Amazon S3 bucket.
{ "Image": { "S3Object": { "Bucket": "bucket", "Name": "inputtext.jpg" } } }
Filters
Filtering by text region, size and confidence score provides you with additional flexibility to control your text detection output. By using regions of interest, you can easily limit text detection to the regions that are relevant to you, for example, the top right of profile photo or a fixed location in relation to a reference point when reading parts numbers from an image of a machine. Word bounding box size filter can be used to avoid small background text which may be noisy or irrelevant. Word confidence filter enables you to remove results that may be unreliable due to being blurry or smudged.
For information regarding filter values, see DetectTextFilters
.
You can use the following filters:
-
MinConfidence –Sets the confidence level of word detection. Words with detection confidence below this level are excluded from the result. Values should be between 0 and 100.
-
MinBoundingBoxWidth – Sets the minimum width of the word bounding box. Words with bounding boxes that are smaller than this value are excluded from the result. The value is relative to the image frame width.
-
MinBoundingBoxHeight – Sets the minimum height of the word bounding box. Words with bounding box heights less than this value are excluded from the result. The value is relative to the image frame height.
-
RegionsOfInterest – Limits detection to a specific region of the image frame. The values are relative to the frame's dimensions. For text only partially within a region, the response is undefined.
DetectText operation response
The DetectText
operation analyzes the image and returns an array,
TextDetections, where each element (TextDetection
) represents a line or word detected in the
image. For each element, DetectText
returns the following information:
-
The detected text (
DetectedText
) -
The relationships between words and lines (
Id
andParentId
) -
The location of text on the image (
Geometry
) -
The confidence Amazon Rekognition has in the accuracy of the detected text and bounding box (
Confidence
) -
The type of the detected text (
Type
)
Detected text
Each TextDetection
element contains recognized text (words or
lines) in the DetectedText
field. A word is one or more script
characters not separated by spaces. DetectText
can detect up to 100
words in an image. Returned text might include characters that make a word
unrecognizable. For example, C@t instead of
Cat. To determine whether a TextDetection
element represents a line of text or a word, use the Type
field.
Each TextDetection
element includes a percentage value that
represents the degree of confidence that Amazon Rekognition has in the accuracy of the
detected text and of the bounding box that surrounds the text.
Word and line relationships
Each TextDetection
element has an identifier field,
Id
. The Id
shows the word's position in a line. If
the element is a word, the parent identifier field, ParentId
,
identifies the line where the word was detected. The ParentId
for a
line is null. For example, the line "but keep" in the example image has the
following the Id
and ParentId
values:
Text |
ID |
Parent ID |
---|---|---|
but keep |
3 |
|
but |
8 |
3 |
keep |
9 |
3 |
Text location on an image
To determine where the recognized text is on an image, use the bounding box
(Geometry)
information that's returned by DetectText
. The
Geometry
object contains two types of bounding box information
for detected lines and words:
-
An axis-aligned coarse rectangular outline in a BoundingBox object
-
A finer-grained polygon that's made up of multiple X and Y coordinates in a Point array
The bounding box and polygon coordinates show where the text is located on the source image. The coordinate values are a ratio of the overall image size. For more information, see BoundingBox.
The following JSON response from the DetectText
operation shows the
words and lines that were detected in the following image.
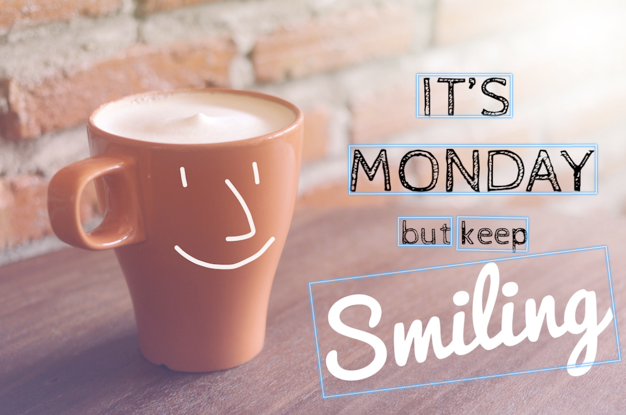
{ 'TextDetections': [{'Confidence': 99.35693359375, 'DetectedText': "IT'S", 'Geometry': {'BoundingBox': {'Height': 0.09988046437501907, 'Left': 0.6684935688972473, 'Top': 0.18226495385169983, 'Width': 0.1461552083492279}, 'Polygon': [{'X': 0.6684935688972473, 'Y': 0.1838926374912262}, {'X': 0.8141663074493408, 'Y': 0.18226495385169983}, {'X': 0.8146487474441528, 'Y': 0.28051772713661194}, {'X': 0.6689760088920593, 'Y': 0.2821454107761383}]}, 'Id': 0, 'Type': 'LINE'}, {'Confidence': 99.6207275390625, 'DetectedText': 'MONDAY', 'Geometry': {'BoundingBox': {'Height': 0.11442459374666214, 'Left': 0.5566731691360474, 'Top': 0.3525116443634033, 'Width': 0.39574965834617615}, 'Polygon': [{'X': 0.5566731691360474, 'Y': 0.353712260723114}, {'X': 0.9522717595100403, 'Y': 0.3525116443634033}, {'X': 0.9524227976799011, 'Y': 0.4657355844974518}, {'X': 0.5568241477012634, 'Y': 0.46693623065948486}]}, 'Id': 1, 'Type': 'LINE'}, {'Confidence': 99.6160888671875, 'DetectedText': 'but keep', 'Geometry': {'BoundingBox': {'Height': 0.08314694464206696, 'Left': 0.6398131847381592, 'Top': 0.5267938375473022, 'Width': 0.2021435648202896}, 'Polygon': [{'X': 0.640289306640625, 'Y': 0.5267938375473022}, {'X': 0.8419567942619324, 'Y': 0.5295097827911377}, {'X': 0.8414806723594666, 'Y': 0.609940767288208}, {'X': 0.6398131847381592, 'Y': 0.6072247624397278}]}, 'Id': 2, 'Type': 'LINE'}, {'Confidence': 88.95134735107422, 'DetectedText': 'Smiling', 'Geometry': {'BoundingBox': {'Height': 0.4326171875, 'Left': 0.46289217472076416, 'Top': 0.5634765625, 'Width': 0.5371078252792358}, 'Polygon': [{'X': 0.46289217472076416, 'Y': 0.5634765625}, {'X': 1.0, 'Y': 0.5634765625}, {'X': 1.0, 'Y': 0.99609375}, {'X': 0.46289217472076416, 'Y': 0.99609375}]}, 'Id': 3, 'Type': 'LINE'}, {'Confidence': 99.35693359375, 'DetectedText': "IT'S", 'Geometry': {'BoundingBox': {'Height': 0.09988046437501907, 'Left': 0.6684935688972473, 'Top': 0.18226495385169983, 'Width': 0.1461552083492279}, 'Polygon': [{'X': 0.6684935688972473, 'Y': 0.1838926374912262}, {'X': 0.8141663074493408, 'Y': 0.18226495385169983}, {'X': 0.8146487474441528, 'Y': 0.28051772713661194}, {'X': 0.6689760088920593, 'Y': 0.2821454107761383}]}, 'Id': 4, 'ParentId': 0, 'Type': 'WORD'}, {'Confidence': 99.6207275390625, 'DetectedText': 'MONDAY', 'Geometry': {'BoundingBox': {'Height': 0.11442466825246811, 'Left': 0.5566731691360474, 'Top': 0.35251158475875854, 'Width': 0.39574965834617615}, 'Polygon': [{'X': 0.5566731691360474, 'Y': 0.3537122905254364}, {'X': 0.9522718787193298, 'Y': 0.35251158475875854}, {'X': 0.9524227976799011, 'Y': 0.4657355546951294}, {'X': 0.5568241477012634, 'Y': 0.46693626046180725}]}, 'Id': 5, 'ParentId': 1, 'Type': 'WORD'}, {'Confidence': 99.96778869628906, 'DetectedText': 'but', 'Geometry': {'BoundingBox': {'Height': 0.0625, 'Left': 0.6402802467346191, 'Top': 0.5283203125, 'Width': 0.08027780801057816}, 'Polygon': [{'X': 0.6402802467346191, 'Y': 0.5283203125}, {'X': 0.7205580472946167, 'Y': 0.5283203125}, {'X': 0.7205580472946167, 'Y': 0.5908203125}, {'X': 0.6402802467346191, 'Y': 0.5908203125}]}, 'Id': 6, 'ParentId': 2, 'Type': 'WORD'}, {'Confidence': 99.26438903808594, 'DetectedText': 'keep', 'Geometry': {'BoundingBox': {'Height': 0.0818721204996109, 'Left': 0.7344760298728943, 'Top': 0.5280686020851135, 'Width': 0.10748066753149033}, 'Polygon': [{'X': 0.7349520921707153, 'Y': 0.5280686020851135}, {'X': 0.8419566750526428, 'Y': 0.5295097827911377}, {'X': 0.8414806127548218, 'Y': 0.6099407076835632}, {'X': 0.7344760298728943, 'Y': 0.6084995269775391}]}, 'Id': 7, 'ParentId': 2, 'Type': 'WORD'}, {'Confidence': 88.95134735107422, 'DetectedText': 'Smiling', 'Geometry': {'BoundingBox': {'Height': 0.4326171875, 'Left': 0.46289217472076416, 'Top': 0.5634765625, 'Width': 0.5371078252792358}, 'Polygon': [{'X': 0.46289217472076416, 'Y': 0.5634765625}, {'X': 1.0, 'Y': 0.5634765625}, {'X': 1.0, 'Y': 0.99609375}, {'X': 0.46289217472076416, 'Y': 0.99609375}]}, 'Id': 8, 'ParentId': 3, 'Type': 'WORD'}], 'TextModelVersion': '3.0'}