本文為英文版的機器翻譯版本,如內容有任何歧義或不一致之處,概以英文版為準。
啟用存留時間 (TTL)
您可以在 Amazon DynamoDB 主控台中啟用 TTL、 AWS Command Line Interface (AWS CLI),或搭配任何假設的開發套件使用 Amazon DynamoDB API 參考。 AWS 在所有分割區上啟用 TTL 大約需要一個小時。
登入 AWS Management Console 並開啟 DynamoDB 支援主控台,網址為 https://console.aws.amazon.com/dynamodb/。
-
選擇 Tables (資料表),然後選擇您想要修改的資料表。
-
在 [其他設定] 索引標籤的 [存留時間 (TTL)] 區段中,選擇 [開啟] 以啟用 TTL。
-
在資料表上啟用 TTL 時,DynamoDB 會要求您識別服務在判斷項目是否符合過期資格時將尋找的特定屬性名稱。TTL 屬性名稱 (如下所示) 區分大小寫,且必須與讀取和寫入作業中定義的屬性相符。不匹配將導致過期的項目被取消刪除。若要重新命名 TTL 屬性,您必須先停用 TTL,然後再重新啟用該屬性,然後再重新啟用該屬性。一旦禁用,TTL 將繼續處理大約 30 分鐘的刪除。必須在還原的資料表上重新設定 TTL。
-
(可選)您可以通過模擬到期日期和時間並匹配一些項目來執行測試。這會為您提供項目的範例清單,並確認有項目包含所提供的 TTL 屬性名稱以及到期時間。
啟用 TTL 之後,當您在 DynamoDB 主控台上檢視項目時,TTL 屬性會標記為 TTL。您可以將滑鼠游標懸停到屬性上,來檢視項目過期的日期和時間。
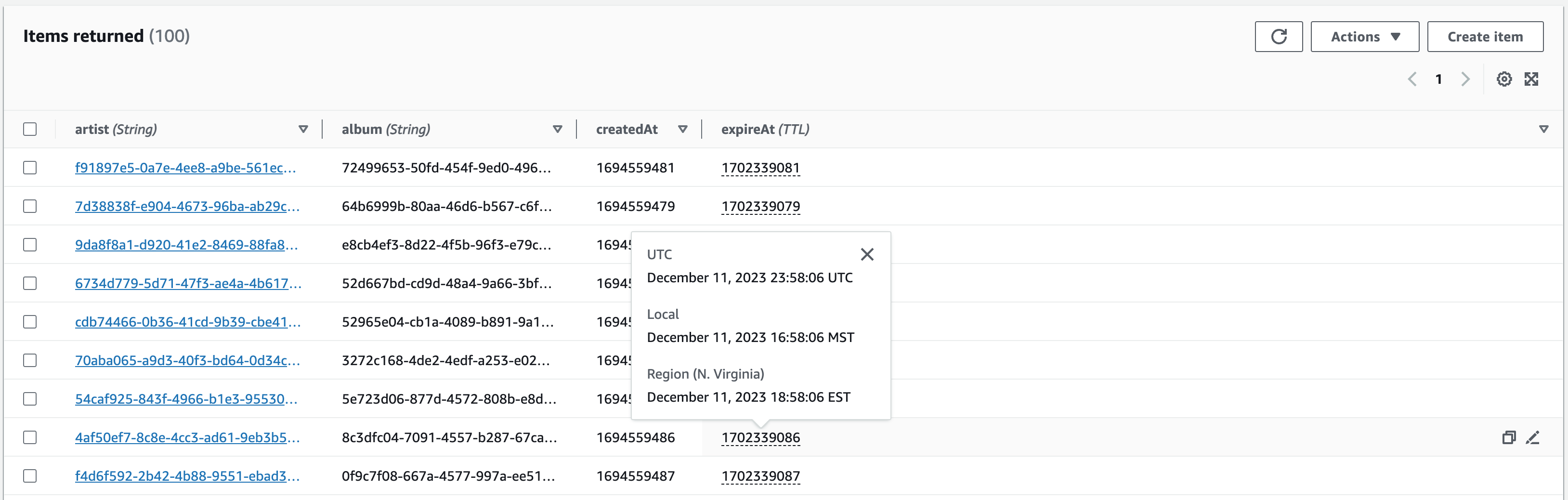
-
啟用
TTLExample
表中的 TTL。aws dynamodb update-time-to-live --table-name TTLExample --time-to-live-specification "Enabled=true, AttributeName=ttl"
-
描述
TTLExample
表中的 TTL。aws dynamodb describe-time-to-live --table-name TTLExample { "TimeToLiveDescription": { "AttributeName": "ttl", "TimeToLiveStatus": "ENABLED" } }
-
使用 BASH shell 及 AWS CLI,將項目新增至已設定存留時間屬性的
TTLExample
資料表。EXP=`date -d '+5 days' +%s` aws dynamodb put-item --table-name "TTLExample" --item '{"id": {"N": "1"}, "ttl": {"N": "'$EXP'"}}'
此範例建立的過期時間為從目前的日期開始加 5 天。然後,將過期時間轉換成 Epoch 時間格式,最終將項目新增到 "TTLExample
" 表。
注意
設定存留時間過期數值的其中一種方式,是計算要新增到過期時間的秒數。例如,5 天等於 432,000 秒。但是通常建議從日期開始操作。
取得目前時間的 Epoch 時間格式非常容易,如以下範例所示。
-
Linux 終端機:
date +%s
-
Python:
import time; int(time.time())
-
Java:
System.currentTimeMillis() / 1000L
-
JavaScript:
Math.floor(Date.now() / 1000)
-
啟用
TTLExample
表中的 TTL。aws dynamodb update-time-to-live --table-name TTLExample --time-to-live-specification "Enabled=true, AttributeName=ttl"
-
描述
TTLExample
表中的 TTL。aws dynamodb describe-time-to-live --table-name TTLExample { "TimeToLiveDescription": { "AttributeName": "ttl", "TimeToLiveStatus": "ENABLED" } }
-
使用 BASH shell 及 AWS CLI,將項目新增至已設定存留時間屬性的
TTLExample
資料表。EXP=`date -d '+5 days' +%s` aws dynamodb put-item --table-name "TTLExample" --item '{"id": {"N": "1"}, "ttl": {"N": "'$EXP'"}}'
此範例建立的過期時間為從目前的日期開始加 5 天。然後,將過期時間轉換成 Epoch 時間格式,最終將項目新增到 "TTLExample
" 表。
注意
設定存留時間過期數值的其中一種方式,是計算要新增到過期時間的秒數。例如,5 天等於 432,000 秒。但是通常建議從日期開始操作。
取得目前時間的 Epoch 時間格式非常容易,如以下範例所示。
-
Linux 終端機:
date +%s
-
Python:
import time; int(time.time())
-
Java:
System.currentTimeMillis() / 1000L
-
JavaScript:
Math.floor(Date.now() / 1000)