Use API Gateway Lambda authorizers
Use a Lambda authorizer (formerly known as a custom authorizer) to control access to your API. When a client makes a request your API's method, API Gateway calls your Lambda authorizer. The Lambda authorizer takes the caller's identity as the input and returns an IAM policy as the output.
Use a Lambda authorizer to implement a custom authorization scheme. Your scheme can use request parameters to determine the caller's identity or use a bearer token authentication strategy such as OAuth or SAML. Create a Lambda authorizer in the API Gateway REST API console, using the AWS CLI, or an AWS SDK.
Lambda authorizer authorization workflow
The following diagram shows the authorization workflow for a Lambda authorizer.
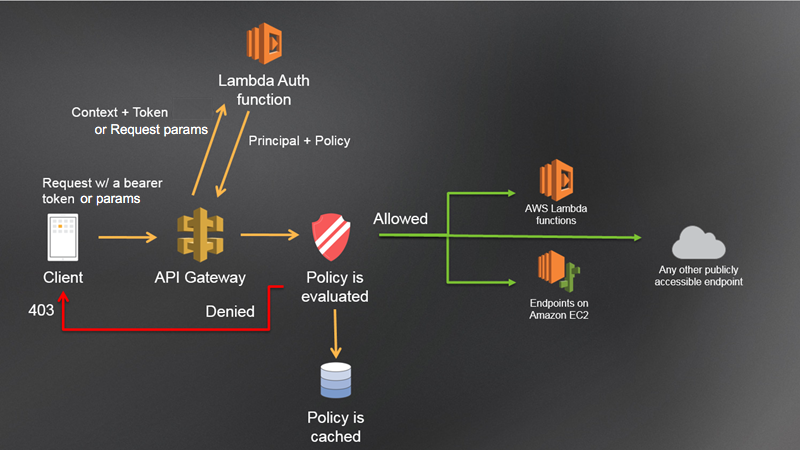
API Gateway Lambda authorization workflow
-
The client calls a method on an API Gateway API, passing a bearer token or request parameters.
-
API Gateway checks if the method request is configured with an Lambda authorizer. If it is, API Gateway calls the Lambda function.
-
The Lambda function authenticates the caller. The function can authenticate in the following ways:
-
By calling out to an OAuth provider to get an OAuth access token.
-
By calling out to a SAML provider to get a SAML assertion.
-
By generating an IAM policy based on the request parameter values.
-
By retrieving credentials from a database.
-
-
The Lambda function returns an IAM policy and a principle identifier.
If the Lambda function does not return that information, the call fails and API Gateway returns a
401 UNAUTHORIZED
HTTP response. -
API Gateway evaluates the IAM policy.
-
If access is denied, API Gateway returns a suitable HTTP status code, such as
403 ACCESS_DENIED
. -
If access is allowed, API Gateway invokes the method.
If you enable authorization caching, API Gateway caches the policy so that the Lambda authorizer function isn't invoked again.
-
You can customize the 403
ACCESS_DENIED
or the 401 UNAUTHORIZED
gateway responses. To learn more, see Gateway responses in API Gateway.
Choosing a type of Lambda authorizer
There are two types of Lambda authorizers:
- Request parameter-based Lambda authorizer (
REQUEST
authorizer) -
A
REQUEST
authorizer receives the caller's identity in a combination of headers, query string parameters, stageVariables, and $context variables. You can use aREQUEST
authorizer to create fine-grained policies based on the information from multiple identity sources, such as the$context.path
and$context.httpMethod
context variables.If you turn on authorization caching for a
REQUEST
authorizer, API Gateway verifies that all specified identity sources are present in the request. If a specified identify source is missing, null, or empty, API Gateway returns a401 Unauthorized
HTTP response without calling the Lambda authorizer function. When multiple identity sources are defined, they are all used to derive the authorizer's cache key, with the order preserved. You can define a fine-grained cache key by using multiple identity sources.If you change any of the cache key parts, and redeploy your API, the authorizer discards the cached policy document and generates a new one.
If you turn off authorization caching for a
REQUEST
authorizer, API Gateway directly passes the request to the Lambda function. - Token-based Lambda authorizer (
TOKEN
authorizer) -
A
TOKEN
authorizer receives the caller's identity in a bearer token, such as a JSON Web Token (JWT) or an OAuth token.If you turn on authorization caching for a
TOKEN
authorizer, the header name specified in the token source becomes the cache key.Additionally, you can use token validation to enter a RegEx statement. API Gateway performs initial validation of the input token against this expression and invokes the Lambda authorizer function upon successful validation. This helps reduce calls to your API.
The
IdentityValidationExpression
property is supported forTOKEN
authorizers only. For more information, see x-amazon-apigateway-authorizer object.
Note
We recommend that you use a REQUEST
authorizer to control access to your API. You can control
access to your API based on multiple identity sources when using a REQUEST
authorizer, compared to
a single identity source when using a TOKEN
authorizer. In addition, you can separate cache keys using
multiple identity sources for a REQUEST
authorizer.
Example REQUEST
authorizer Lambda function
The following example code creates a Lambda authorizer function that allows a request if the client-supplied
headerauth1
header, QueryString1
query parameter, and stage variable of
StageVar1
all match the specified values of headerValue1
, queryValue1
, and
stageValue1
, respectively.
In this example, the Lambda authorizer function checks the input parameters and acts as follows:
-
If all the required parameter values match the expected values, the authorizer function returns a
200 OK
HTTP response and an IAM policy that looks like the following, and the method request succeeds:{ "Version": "2012-10-17", "Statement": [ { "Action": "execute-api:Invoke", "Effect": "Allow", "Resource": "arn:aws:execute-api:us-east-1:123456789012:ivdtdhp7b5/ESTestInvoke-stage/GET/" } ] }
-
Otherwise, the authorizer function returns a
401 Unauthorized
HTTP response, and the method request fails.
In addition to returning an IAM policy, the Lambda authorizer function must also return the caller's
principal identifier. Optionally, it can return a context
object containing additional
information that can be passed into the integration backend. For more information, see Output from an Amazon API Gateway Lambda authorizer.
In production code, you might need to authenticate the user before granting authorization. You can add authentication logic in the Lambda function by calling an authentication provider as directed in the documentation for that provider.
Example TOKEN
authorizer
Lambda function
The following example code creates a TOKEN
Lambda authorizer function that allows a caller to invoke a
method if the client-supplied token value is allow
. The caller is not allowed to invoke the request if the token
value is deny
. If the token value is
unauthorized
or an empty string, the authorizer function returns an 401 UNAUTHORIZED
response.
In this example, when the API receives a method request, API Gateway passes the source token to this Lambda
authorizer function in the event.authorizationToken
attribute. The Lambda authorizer function reads
the token and acts as follows:
-
If the token value is
allow
, the authorizer function returns a200 OK
HTTP response and an IAM policy that looks like the following, and the method request succeeds:{ "Version": "2012-10-17", "Statement": [ { "Action": "execute-api:Invoke", "Effect": "Allow", "Resource": "arn:aws:execute-api:us-east-1:123456789012:ivdtdhp7b5/ESTestInvoke-stage/GET/" } ] }
-
If the token value is
deny
, the authorizer function returns a200 OK
HTTP response and aDeny
IAM policy that looks like the following, and the method request fails:{ "Version": "2012-10-17", "Statement": [ { "Action": "execute-api:Invoke", "Effect": "Deny", "Resource": "arn:aws:execute-api:us-east-1:123456789012:ivdtdhp7b5/ESTestInvoke-stage/GET/" } ] }
Note
Outside of the test environment, API Gateway returns a
403 Forbidden
HTTP response and the method request fails. -
If the token value is
unauthorized
or an empty string, the authorizer function returns a401 Unauthorized
HTTP response, and the method call fails. -
If the token is anything else, the client receives a
500 Invalid token
response, and the method call fails.
In addition to returning an IAM policy, the Lambda authorizer function must also return the caller's
principal identifier. Optionally, it can return a context
object containing additional
information that can be passed into the integration backend. For more information, see Output from an Amazon API Gateway Lambda authorizer.
In production code, you might need to authenticate the user before granting authorization. You can add authentication logic in the Lambda function by calling an authentication provider as directed in the documentation for that provider.
Additional examples of Lambda authorizer functions
The following list shows additional examples of Lambda authorizer functions. You can create a Lambda function in the same account, or a different account, from where you created your API.
For the previous example Lambda functions, you can use the built-in AWSLambdaBasicExecutionRole, as these functions don't call other AWS services. If your Lambda function calls other AWS services, you'll need to assign an IAM execution role to the Lambda function. To create the role, follow the instructions in AWS Lambda Execution Role.
Additional example Lambda authorizer functions
-
For an example application, see Open Banking Brazil - Authorization Samples
on GitHub. -
For more example Lambda functions, see aws-apigateway-lambda-authorizer-blueprints
on GitHub. -
You can create a Lambda authorizer that authenticates users using Amazon Cognito user pools and authorizes callers based on a policy store using Verified Permissions. For more information, see Create a policy store with a connected API and identity provider in the Amazon Verified Permissions User Guide.
-
The Lambda console provides a Python blueprint, which you can use by choosing Use a blueprint and choosing the api-gateway-authorizer-python blueprint.