Mapping templates for REST APIs
In API Gateway, an API's method request or response can take a payload in a different format from the integration request or response.
You can transform your data to:
Match the payload to an API-specified format.
Override an API's request and response parameters and status codes.
Return client selected response headers.
Associate path parameters, query string parameters, or header parameters in the method request of HTTP proxy or AWS service proxy.
Select which data to send using integration with AWS services, such as Amazon DynamoDB or Lambda functions, or HTTP endpoints.
You can use mapping templates to transform your data. A mapping template is a script
expressed in Velocity Template Language
(VTL)
This section describes conceptual information related to mapping templates. For instructions on creating mapping template for an API Gateway REST API, see Set up data transformations in API Gateway.
Mapping template example
The following example is input data to an integration request.
[ { "id": 1, "type": "dog", "price": 249.99 }, { "id": 2, "type": "cat", "price": 124.99 }, { "id": 3, "type": "fish", "price": 0.99 } ]
The following example is a mapping template to transform the integration request data.
#set($inputRoot = $input.path('$')) [ #foreach($elem in $inputRoot) { "description" : "Item $elem.id is a $elem.type.", "askingPrice" : $elem.price }#if($foreach.hasNext),#end #end ]
The following example is output data from the transformation.
[ { "description" : "Item 1 is a dog.", "askingPrice" : 249.99 }, { "description" : "Item 2 is a cat.", "askingPrice" : 124.99 }, { "description" : "Item 3 is a fish.", "askingPrice" : 0.99 } ]
The following diagram shows details of this mapping template.
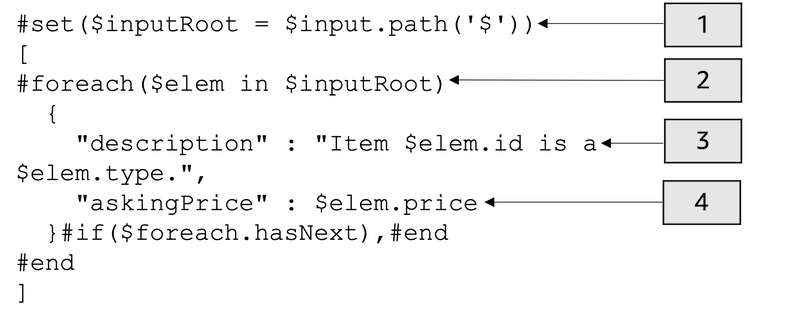
The
$inputRoot
variable represents the root object in the original JSON data from the previous section. Directives begin with the#
symbol.A
foreach
loop iterates though each object in the original JSON data.The description is a concatenation of the Pet's
id
andtype
from the original JSON data.askingPrice
is theprice
is the price from the original JSON data.
1 #set($inputRoot = $input.path('$')) 2 [ 3 #foreach($elem in $inputRoot) 4 { 5 "description" : "Item $elem.id is a $elem.type.", 6 "askingPrice" : $elem.price 7 }#if($foreach.hasNext),#end 8 #end 9 ]
In this mapping template:
-
On line 1, the
$inputRoot
variable represents the root object in the original JSON data from the previous section. Directives begin with the#
symbol. -
On line 3, a
foreach
loop iterates through each object in the original JSON data. -
On line 5, the
description
is a concatenation of the Pet'sid
andtype
from the original JSON data. -
On line 6,
askingPrice
is theprice
is the price from the original JSON data.
For more information about the Velocity Template Language, see Apache
Velocity - VTL Reference
The mapping template assumes that the underlying data is of a JSON object. It does not require that a model be defined for the data. However, a model for the output data allows preceding data to be returned as a language-specific object. For more information, see Data models for REST APIs.
Complex mapping template examples
You can also create more complicated mapping templates. The following example shows the concatenation of references and a cutoff of 100 to determine if a pet is affordable.
The following example is input data to an integration request.
[ { "id": 1, "type": "dog", "price": 249.99 }, { "id": 2, "type": "cat", "price": 124.99 }, { "id": 3, "type": "fish", "price": 0.99 } ]
The following example is a mapping template to transform the integration request data.
#set($inputRoot = $input.path('$')) #set($cheap = 100) [ #foreach($elem in $inputRoot) { #set($name = "${elem.type}number$elem.id") "name" : $name, "description" : "Item $elem.id is a $elem.type.", #if($elem.price > $cheap )#set ($afford = 'too much!') #{else}#set ($afford = $elem.price)#end "askingPrice" : $afford }#if($foreach.hasNext),#end #end ]
The following example is output data from the transformation.
[ { "name" : dognumber1, "description" : "Item 1 is a dog.", "askingPrice" : too much! }, { "name" : catnumber2, "description" : "Item 2 is a cat.", "askingPrice" : too much! }, { "name" : fishnumber3, "description" : "Item 3 is a fish.", "askingPrice" : 0.99 } ]
You can also see more complicated data models. See Example data models and mapping templates for API Gateway.