本文属于机器翻译版本。若本译文内容与英语原文存在差异,则一律以英文原文为准。
AWS DeepRacer 奖励函数的输入参数
AWS DeepRacer 奖励函数将字典对象作为输入。
def reward_function(params) : reward = ... return float(reward)
params
词典对象包含以下键/值对:
{ "all_wheels_on_track": Boolean, # flag to indicate if the agent is on the track "x": float, # agent's x-coordinate in meters "y": float, # agent's y-coordinate in meters "closest_objects": [int, int], # zero-based indices of the two closest objects to the agent's current position of (x, y). "closest_waypoints": [int, int], # indices of the two nearest waypoints. "distance_from_center": float, # distance in meters from the track center "is_crashed": Boolean, # Boolean flag to indicate whether the agent has crashed. "is_left_of_center": Boolean, # Flag to indicate if the agent is on the left side to the track center or not. "is_offtrack": Boolean, # Boolean flag to indicate whether the agent has gone off track. "is_reversed": Boolean, # flag to indicate if the agent is driving clockwise (True) or counter clockwise (False). "heading": float, # agent's yaw in degrees "objects_distance": [float, ], # list of the objects' distances in meters between 0 and track_length in relation to the starting line. "objects_heading": [float, ], # list of the objects' headings in degrees between -180 and 180. "objects_left_of_center": [Boolean, ], # list of Boolean flags indicating whether elements' objects are left of the center (True) or not (False). "objects_location": [(float, float),], # list of object locations [(x,y), ...]. "objects_speed": [float, ], # list of the objects' speeds in meters per second. "progress": float, # percentage of track completed "speed": float, # agent's speed in meters per second (m/s) "steering_angle": float, # agent's steering angle in degrees "steps": int, # number steps completed "track_length": float, # track length in meters. "track_width": float, # width of the track "waypoints": [(float, float), ] # list of (x,y) as milestones along the track center }
输入参数的更多详细技术参考如下所示。
all_wheels_on_track
类型:Boolean
范围:(True:False)
一个 Boolean
标记,指示代理是在赛道上还是偏离赛道。如果车辆的任一车轮位于赛道边界外,则将车辆视为偏离赛道 (False
)。如果车辆的所有车轮都在两个赛道边界内,则将车辆视为在赛道上 (True
)。下图显示了代理在赛道上。
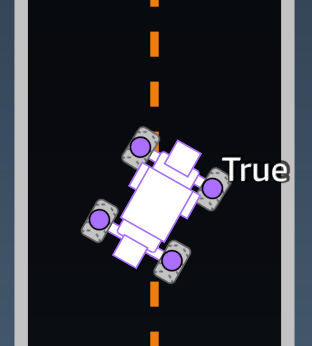
下图显示了代理偏离赛道。
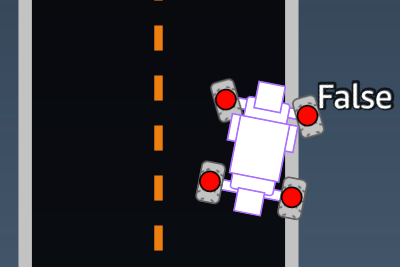
示例:使用 all_wheels_on_track
参数的奖励函数
def reward_function(params): ############################################################################# ''' Example of using all_wheels_on_track and speed ''' # Read input variables all_wheels_on_track = params['all_wheels_on_track'] speed = params['speed'] # Set the speed threshold based your action space SPEED_THRESHOLD = 1.0 if not all_wheels_on_track: # Penalize if the car goes off track reward = 1e-3 elif speed < SPEED_THRESHOLD: # Penalize if the car goes too slow reward = 0.5 else: # High reward if the car stays on track and goes fast reward = 1.0 return float(reward)
closest_waypoints
类型:[int, int]
范围:[(0:Max-1),(1:Max-1)]
最接近代理当前位置 (x, y)
的两个相邻 waypoint
的从零开始的索引。距离是根据与代理中心的欧氏距离来测量的。第一个元素指代理后面最近的路点,第二个元素指代理前面最近的路点。Max
是路点列表的长度。在waypoints的图示中,closest_waypoints
将为 [16, 17]
。
示例:使用 closest_waypoints
参数的奖励函数。
以下示例奖励函数演示如何使用 waypoints
、closest_waypoints
和 heading
来计算即时奖励。
AWS DeepRacer 支持以下库:数学、随机 NumPy SciPy、和 Shapely。要使用一个库,请在函数定义 def function_name(parameters)
的上方添加一个 import 语句 import
。supported library
# Place import statement outside of function (supported libraries: math, random, numpy, scipy, and shapely) # Example imports of available libraries # # import math # import random # import numpy # import scipy # import shapely import math def reward_function(params): ############################################################################### ''' Example of using waypoints and heading to make the car point in the right direction ''' # Read input variables waypoints = params['waypoints'] closest_waypoints = params['closest_waypoints'] heading = params['heading'] # Initialize the reward with typical value reward = 1.0 # Calculate the direction of the center line based on the closest waypoints next_point = waypoints[closest_waypoints[1]] prev_point = waypoints[closest_waypoints[0]] # Calculate the direction in radius, arctan2(dy, dx), the result is (-pi, pi) in radians track_direction = math.atan2(next_point[1] - prev_point[1], next_point[0] - prev_point[0]) # Convert to degree track_direction = math.degrees(track_direction) # Calculate the difference between the track direction and the heading direction of the car direction_diff = abs(track_direction - heading) if direction_diff > 180: direction_diff = 360 - direction_diff # Penalize the reward if the difference is too large DIRECTION_THRESHOLD = 10.0 if direction_diff > DIRECTION_THRESHOLD: reward *= 0.5 return float(reward)
closest_objects
类型:[int, int]
范围:[(0:len(objects_location)-1),
(0:len(objects_location)-1)]
最接近代理当前位置 (x, y) 的两个物体的从零开始的索引。第一个索引指代理后面最近的物体,第二个索引指代理前面最近的物体。如果只有一个物体,则两个索引都为 0。
distance_from_center
类型:float
范围:0:~track_width/2
代理中心和赛道中心之间的位移(以米为单位)。当代理的任一车轮位于赛道边界外时可观察到的最大位移,并且根据赛道边界的宽度,它可以略小于或大于 track_width
的一半。
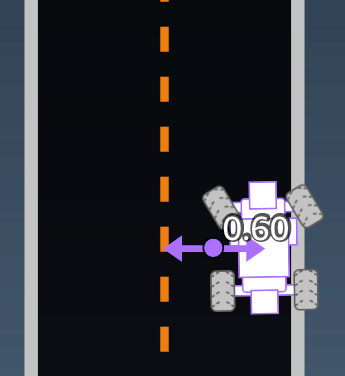
示例:使用 distance_from_center
参数的奖励函数
def reward_function(params): ################################################################################# ''' Example of using distance from the center ''' # Read input variable track_width = params['track_width'] distance_from_center = params['distance_from_center'] # Penalize if the car is too far away from the center marker_1 = 0.1 * track_width marker_2 = 0.5 * track_width if distance_from_center <= marker_1: reward = 1.0 elif distance_from_center <= marker_2: reward = 0.5 else: reward = 1e-3 # likely crashed/ close to off track return float(reward)
heading
类型:float
范围:-180:+180
代理相对于坐标系 x 轴的前进方向(以度为单位)。
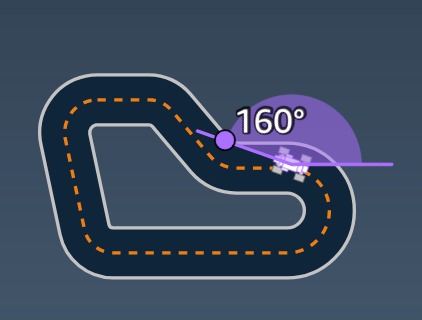
示例:使用 heading
参数的奖励函数
有关更多信息,请参阅 closest_waypoints。
is_crashed
类型:Boolean
范围:(True:False)
一个布尔标记,用于指示代理的最终状态是否为撞向另一个物体(True
或 False
)。
is_left_of_center
类型:Boolean
范围:[True : False]
一个 Boolean
标记,用于指示代理是位于赛道中心的左侧 (True
) 还是右侧 (False
)。
is_offtrack
类型:Boolean
范围:(True:False)
一个布尔标记,用于指示代理的最终状态是否为脱离赛道(True 或 False)。
is_reversed
类型:Boolean
范围:[True:False]
一个布尔标记,用于指示代理是顺时针行驶 (True) 还是逆时针行驶 (False)。
此参数在您针对每个过程改变方向时使用。
objects_distance
类型:[float, … ]
范围:[(0:track_length), … ]
环境中物体之间相对于起始线的距离列表。第 i 个元素测量沿赛道中心线第 i 个物体与起始线之间的距离。
注意
abs | (var1) - (var2)| = 汽车与物体的接近程度, WHEN var1 = ["objects_distance"][index] and var2 = params["progress"]*params["track_length"]
要获取车辆前方最近物体和车辆后方最近物体的索引,请使用“closest_objects”参数。
objects_heading
类型:[float, … ]
范围:[(-180:180), … ]
物体前进方向(以度为单位)列表。第 i 个元素测量第 i 个物体的前进方向。对于静止物体,其前进方向为 0。对于自动程序车辆,相应元素的值是车辆的前进角度。
objects_left_of_center
类型:[Boolean, … ]
范围:[True|False, … ]
布尔标记列表。第 i 个元素值指示第 i 个物体位于赛道中心的左侧 (True) 还是右侧 (False)。
objects_location
类型:[(x,y), … ]
范围:[(0:N,0:N), … ]
所有物体位置的列表,每个位置都是 (x, y) 的元组。
列表的大小等于赛道上的物体数。注意,物体可能是静止的障碍物,移动的自动程序车辆。
objects_speed
类型:[float, … ]
范围:[(0:12.0), … ]
赛道上物体的速度(米/秒)列表。对于静止物体,其速度为 0。对于自动程序车辆,值为您在训练中设置的速度。
进度
类型:float
范围:0:100
赛道完成百分比。
示例:使用 progress
参数的奖励函数
有关更多信息,请参阅步骤。
speed
类型:float
范围:0.0:5.0
观察到的代理速度,以米/秒 (m/s) 为单位。
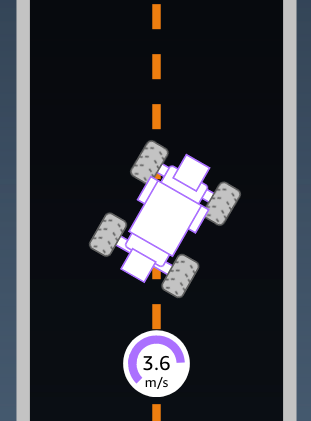
示例:使用 speed
参数的奖励函数
有关更多信息,请参阅 all_wheels_on_track。
steering_angle
类型:float
范围:-30:30
前轮与代理中心线之间的转向角(以度为单位)。负号 (-) 表示向右转向,正号 (+) 表示向左转向。代理中心线不一定与赛道中心线平行,如下图所示。
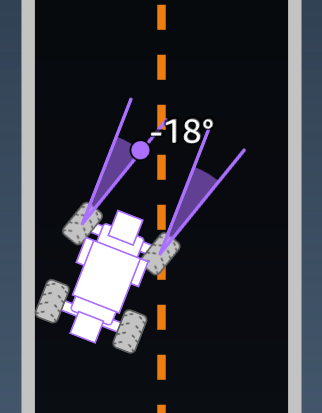
示例:使用 steering_angle
参数的奖励函数
def reward_function(params): ''' Example of using steering angle ''' # Read input variable abs_steering = abs(params['steering_angle']) # We don't care whether it is left or right steering # Initialize the reward with typical value reward = 1.0 # Penalize if car steer too much to prevent zigzag ABS_STEERING_THRESHOLD = 20.0 if abs_steering > ABS_STEERING_THRESHOLD: reward *= 0.8 return float(reward)
步骤
类型:int
范围:0:Nstep
完成的步骤数。步骤对应于代理按照当前策略所采取的操作。
示例:使用 steps
参数的奖励函数
def reward_function(params): ############################################################################# ''' Example of using steps and progress ''' # Read input variable steps = params['steps'] progress = params['progress'] # Total num of steps we want the car to finish the lap, it will vary depends on the track length TOTAL_NUM_STEPS = 300 # Initialize the reward with typical value reward = 1.0 # Give additional reward if the car pass every 100 steps faster than expected if (steps % 100) == 0 and progress > (steps / TOTAL_NUM_STEPS) * 100 : reward += 10.0 return float(reward)
track_length
类型:float
范围:[0:Lmax]
赛道长度(以米为单位)。Lmax is track-dependent.
track_width
类型:float
范围:0:Dtrack
赛道宽度(以米为单位)。
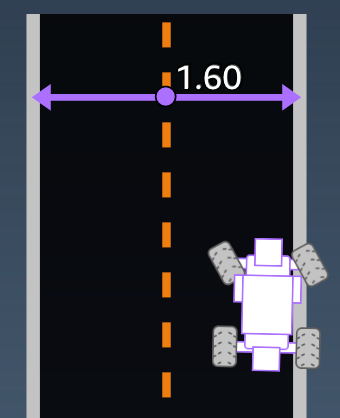
示例:使用 track_width
参数的奖励函数
def reward_function(params): ############################################################################# ''' Example of using track width ''' # Read input variable track_width = params['track_width'] distance_from_center = params['distance_from_center'] # Calculate the distance from each border distance_from_border = 0.5 * track_width - distance_from_center # Reward higher if the car stays inside the track borders if distance_from_border >= 0.05: reward = 1.0 else: reward = 1e-3 # Low reward if too close to the border or goes off the track return float(reward)
x, y
类型:float
范围:0:N
包含赛道的模拟环境的沿 x 和 y 轴的代理中心位置(以米为单位)。原点位于模拟环境的左下角。
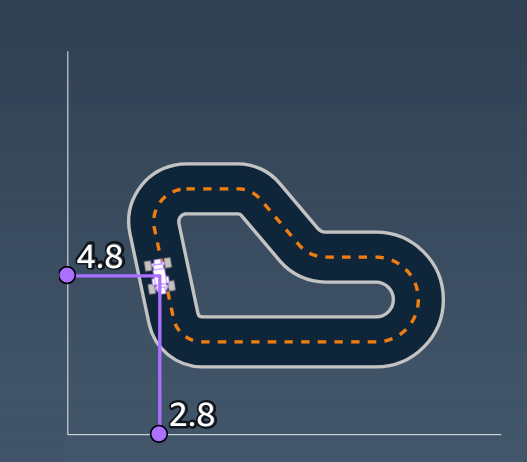
waypoints
类型:[float, float]
的 list
范围:[[xw,0,yw,0] …
[xw,Max-1,
yw,Max-1]]
沿赛道中心排列、取决于赛道的 Max
里程的有序列表。每个里程碑均由 (xw,i, yw,i) 坐标描述。对于环形赛道,第一个路径点与最后一个路径点相同。对于直道和其他非环形赛道,第一个路径点与最后一个路径点不同。
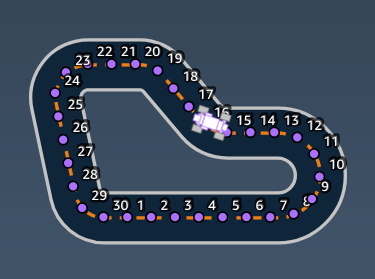
示例:使用 waypoints
参数的奖励函数
有关更多信息,请参阅 closest_waypoints。