本文為英文版的機器翻譯版本,如內容有任何歧義或不一致之處,概以英文版為準。
AWS DeepRacer 獎勵功能的輸入參數
AWS DeepRacer 獎勵函數需要一個字典對象作為輸入。
def reward_function(params) : reward = ... return float(reward)
params
字典物件包含下列鍵/值對:
{ "all_wheels_on_track": Boolean, # flag to indicate if the agent is on the track "x": float, # agent's x-coordinate in meters "y": float, # agent's y-coordinate in meters "closest_objects": [int, int], # zero-based indices of the two closest objects to the agent's current position of (x, y). "closest_waypoints": [int, int], # indices of the two nearest waypoints. "distance_from_center": float, # distance in meters from the track center "is_crashed": Boolean, # Boolean flag to indicate whether the agent has crashed. "is_left_of_center": Boolean, # Flag to indicate if the agent is on the left side to the track center or not. "is_offtrack": Boolean, # Boolean flag to indicate whether the agent has gone off track. "is_reversed": Boolean, # flag to indicate if the agent is driving clockwise (True) or counter clockwise (False). "heading": float, # agent's yaw in degrees "objects_distance": [float, ], # list of the objects' distances in meters between 0 and track_length in relation to the starting line. "objects_heading": [float, ], # list of the objects' headings in degrees between -180 and 180. "objects_left_of_center": [Boolean, ], # list of Boolean flags indicating whether elements' objects are left of the center (True) or not (False). "objects_location": [(float, float),], # list of object locations [(x,y), ...]. "objects_speed": [float, ], # list of the objects' speeds in meters per second. "progress": float, # percentage of track completed "speed": float, # agent's speed in meters per second (m/s) "steering_angle": float, # agent's steering angle in degrees "steps": int, # number steps completed "track_length": float, # track length in meters. "track_width": float, # width of the track "waypoints": [(float, float), ] # list of (x,y) as milestones along the track center }
輸入參數的更詳細技術參考如下。
all_wheels_on_track
Type (類型):Boolean
Range (範圍):(True:False)
Boolean
標記,指出代理程式是在賽道上,還是脫離賽道。若它有任何一個輪胎位於軌道邊界外側,便會視為脫離軌道 (False
)。若所有輪胎皆位於兩個軌道邊界內,則為位於軌道上 (True
)。下圖顯示代理程式正在賽道上。
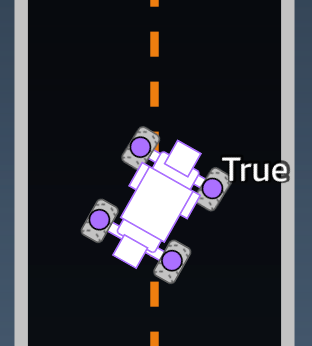
下圖顯示代理程式脫離賽道。
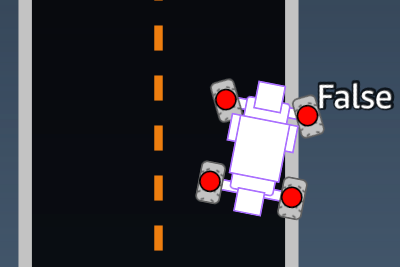
範例:使用all_wheels_on_track
參數的獎勵函式
def reward_function(params): ############################################################################# ''' Example of using all_wheels_on_track and speed ''' # Read input variables all_wheels_on_track = params['all_wheels_on_track'] speed = params['speed'] # Set the speed threshold based your action space SPEED_THRESHOLD = 1.0 if not all_wheels_on_track: # Penalize if the car goes off track reward = 1e-3 elif speed < SPEED_THRESHOLD: # Penalize if the car goes too slow reward = 0.5 else: # High reward if the car stays on track and goes fast reward = 1.0 return float(reward)
closest_waypoints
Type (類型):[int, int]
Range (範圍):[(0:Max-1),(1:Max-1)]
兩個與代理程式目前位置 ((x, y)
) 最接近相鄰 waypoint
以零為基礎的索引。距離會以從代理程式中心計算的歐幾里得距離測量。第一個元素是代理程式後方最接近的導航點,第二個元素則是代理程式前方最接近的導航點。Max
則是導航點清單的長度。在 waypoints 中顯示的圖例中,closest_waypoints
會是 [16, 17]
。
Example (範例):使用 closest_waypoints
參數的獎勵函數。
以下範例獎勵函數會示範如何使用 waypoints
和 closest_waypoints
,以及 heading
來計算立即性獎勵。
AWS DeepRacer 支援下列程式庫:數學、隨機 NumPy、 SciPy、和美麗。若要使用一個,請在函數定義上方新增 import 陳述式def function_name(parameters)
。import
supported library
# Place import statement outside of function (supported libraries: math, random, numpy, scipy, and shapely) # Example imports of available libraries # # import math # import random # import numpy # import scipy # import shapely import math def reward_function(params): ############################################################################### ''' Example of using waypoints and heading to make the car point in the right direction ''' # Read input variables waypoints = params['waypoints'] closest_waypoints = params['closest_waypoints'] heading = params['heading'] # Initialize the reward with typical value reward = 1.0 # Calculate the direction of the center line based on the closest waypoints next_point = waypoints[closest_waypoints[1]] prev_point = waypoints[closest_waypoints[0]] # Calculate the direction in radius, arctan2(dy, dx), the result is (-pi, pi) in radians track_direction = math.atan2(next_point[1] - prev_point[1], next_point[0] - prev_point[0]) # Convert to degree track_direction = math.degrees(track_direction) # Calculate the difference between the track direction and the heading direction of the car direction_diff = abs(track_direction - heading) if direction_diff > 180: direction_diff = 360 - direction_diff # Penalize the reward if the difference is too large DIRECTION_THRESHOLD = 10.0 if direction_diff > DIRECTION_THRESHOLD: reward *= 0.5 return float(reward)
closest_objects
Type (類型):[int, int]
Range (範圍):[(0:len(objects_location)-1),
(0:len(objects_location)-1)]
兩個與代理程式目前位置 (x, y) 最接近物件的以零為基礎索引。第一個索引是代理程式後方最接近的物件,第二個索引則是代理程式前方最接近的物件。如果只有一個物件,則兩個索引都將會是 0。
distance_from_center
Type (類型):float
Range (範圍):0:~track_width/2
代理程式中心及賽道中心之間的偏移 (公尺)。可觀察到的最大偏移會在代理程式的任何一個輪胎位於軌道邊界外圍時發生,取決於軌道邊界的寬度,這可能會略小或略大於 track_width
的一半。
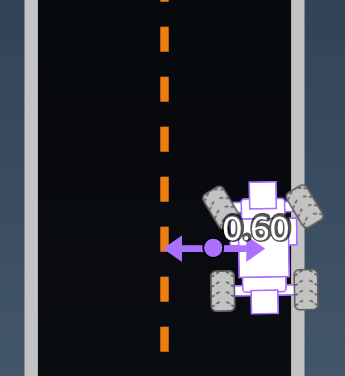
範例:使用distance_from_center
參數的獎勵函式
def reward_function(params): ################################################################################# ''' Example of using distance from the center ''' # Read input variable track_width = params['track_width'] distance_from_center = params['distance_from_center'] # Penalize if the car is too far away from the center marker_1 = 0.1 * track_width marker_2 = 0.5 * track_width if distance_from_center <= marker_1: reward = 1.0 elif distance_from_center <= marker_2: reward = 0.5 else: reward = 1e-3 # likely crashed/ close to off track return float(reward)
heading
Type (類型):float
Range (範圍):-180:+180
代理程式的車頭方向 (角度),相對於座標系統的 X 軸。
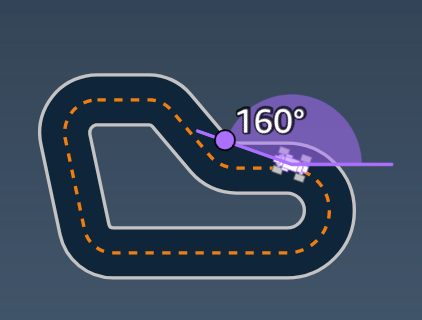
範例:使用heading
參數的獎勵函式
如需詳細資訊,請參閱 closest_waypoints。
is_crashed
Type (類型):Boolean
Range (範圍):(True:False)
布林標記,指出做為代理程式的終止狀態,代理程式與另外一個物件發生衝撞 (True
) 還是沒有發生衝撞 (False
)。
is_left_of_center
Type (類型):Boolean
Range (範圍):[True : False]
Boolean
標記,指出代理程式位於賽道中心的左側 (True
) 還是右側 (False
)。
is_offtrack
Type (類型):Boolean
Range (範圍):(True:False)
布林標記,指出做為代理程式的終止狀態,代理程式脫離了賽道 (True) 還是沒有 (False)。
is_reversed
Type (類型):Boolean
Range (範圍):[True:False]
布林標記,指出代理程式正以順時針方向駕駛 (True) 還是逆時針方向 (False)。
此標記會在您為每個回合啟用方向變更時使用。
objects_distance
Type (類型):[float, … ]
Range (範圍):[(0:track_length), … ]
環境中物件之間相對於起始線之間的距離清單。第 i 個元素會測量沿著賽道中心線,第 i 個物件與起始線之間的距離 (公尺)。
注意
abs | (var1) - (var2)| = how close the car is to an object, WHEN var1 = ["objects_distance"][index] and var2 = params["progress"]*params["track_length"]
若要取得車輛前方最近物體的索引,以及車輛後方最近物體的索引,請使用「closest_object」參數。
objects_heading
Type (類型):[float, … ]
Range (範圍):[(-180:180), … ]
物件所面對方向的清單 (角度)。第 i 個元素會測量第 i 個物件所面對的方向。針對固定物件,面對方向的角度為 0。針對機器人車輛,對應元素的值是車輛車頭的角度。
objects_left_of_center
Type (類型):[Boolean, … ]
Range (範圍):[True|False, … ]
布林標記清單。第 i 個元素值指出第 i 個物件是在賽道中心的左側 (True) 還是右側 (False)。
objects_location
Type (類型):[(x,y), … ]
Range (範圍):[(0:N,0:N), … ]
所有物件的位置清單,每個位置都是 (x, y) 的元組。
清單的大小與賽道上的物件數量相等。請注意,物件可能會是固定的障礙物或是正在移動的機器人車輛。
objects_speed
Type (類型):[float, … ]
Range (範圍):[(0:12.0), … ]
賽道上物件的速度清單 (單位為每秒的公尺數)。針對固定物件,其速度為 0。對於機器人車輛而言,該值是您在訓練中設定的速度。
進度
Type (類型):float
Range (範圍):0:100
完成軌道的百分比。
範例:使用progress
參數的獎勵函式
如需詳細資訊,請參閱梯度。
speed
Type (類型):float
Range (範圍):0.0:5.0
代理程式所觀察到的速度,單位為公尺/每秒 (m/s)。
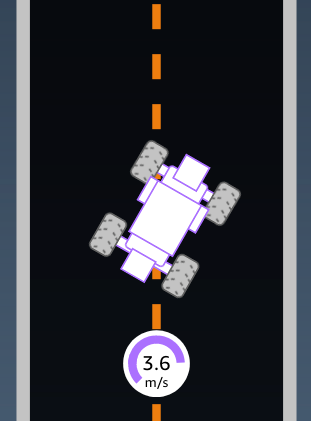
範例:使用speed
參數的獎勵函式
如需詳細資訊,請參閱 all_wheels_on_track。
steering_angle
Type (類型):float
Range (範圍):-30:30
前輪偏移代理程式中線的方向盤角度 (度)。負號 (-) 表示向右偏移,正號 (+) 表示向左偏移。代理程式中線不見得會和賽道中線平行,如下圖所示。
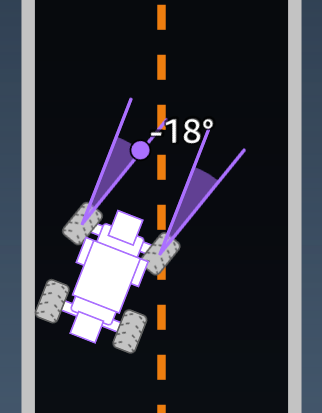
範例:使用steering_angle
參數的獎勵函式
def reward_function(params): ''' Example of using steering angle ''' # Read input variable abs_steering = abs(params['steering_angle']) # We don't care whether it is left or right steering # Initialize the reward with typical value reward = 1.0 # Penalize if car steer too much to prevent zigzag ABS_STEERING_THRESHOLD = 20.0 if abs_steering > ABS_STEERING_THRESHOLD: reward *= 0.8 return float(reward)
steps
Type (類型):int
Range (範圍):0:Nstep
完成的步驟數。步驟對應到遵循目前政策代理程式所採取的動作。
範例:使用steps
參數的獎勵函式
def reward_function(params): ############################################################################# ''' Example of using steps and progress ''' # Read input variable steps = params['steps'] progress = params['progress'] # Total num of steps we want the car to finish the lap, it will vary depends on the track length TOTAL_NUM_STEPS = 300 # Initialize the reward with typical value reward = 1.0 # Give additional reward if the car pass every 100 steps faster than expected if (steps % 100) == 0 and progress > (steps / TOTAL_NUM_STEPS) * 100 : reward += 10.0 return float(reward)
track_length
Type (類型):float
Range (範圍):[0:Lmax]
以公尺為單位的賽道長度。Lmax is track-dependent.
track_width
Type (類型):float
Range (範圍):0:Dtrack
軌道寬度 (公尺)。
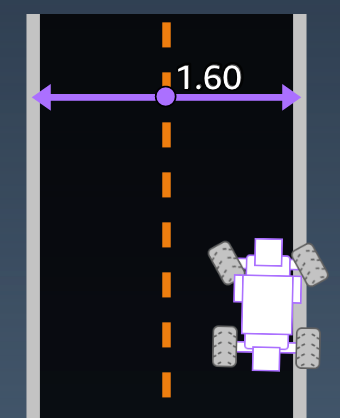
範例:使用track_width
參數的獎勵函式
def reward_function(params): ############################################################################# ''' Example of using track width ''' # Read input variable track_width = params['track_width'] distance_from_center = params['distance_from_center'] # Calculate the distance from each border distance_from_border = 0.5 * track_width - distance_from_center # Reward higher if the car stays inside the track borders if distance_from_border >= 0.05: reward = 1.0 else: reward = 1e-3 # Low reward if too close to the border or goes off the track return float(reward)
x, y
Type (類型):float
Range (範圍):0:N
代理程式中心在包含賽道模擬環境中沿著 x 和 y 軸的位置 (公尺)。原點位於模擬環境的左下角。
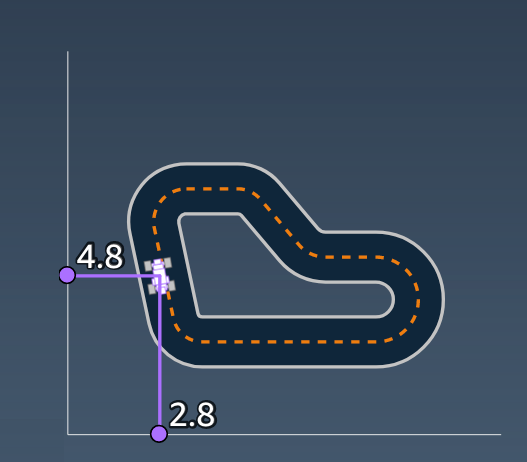
waypoints
Type (類型):[float, float]
的 list
Range (範圍):[[xw,0,yw,0] …
[xw,Max-1,
yw,Max-1]]
沿著賽道中心的相依賽道 Max
里程碑排序清單。每個里程碑由 (xw,i, yw,i) 的座標來描述。對於環狀軌道,第一個和最後一個路徑點是相同的。對於直線軌道或其他非環狀軌道,第一個和最後一個路徑點則不同。
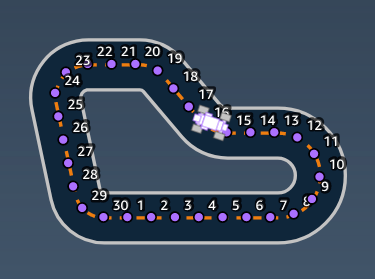
示例使用參數的獎勵函waypoints
數
如需更多詳細資訊,請參閱 closest_waypoints。