Managing resource permissions with AWS SAM connectors
Topics
What are AWS SAM connectors?
Connectors are an AWS Serverless Application Model (AWS SAM) abstract resource type, identified as
AWS::Serverless::Connector
, that provides simple and well-scoped permissions between your serverless
application resources. Use the Connectors
resource attribute by embedding it within a
source resource. Then, define your destination
resource and describe how data or events should flow between those resources. AWS SAM then composes the access policies
necessary to facilitate the required interactions.
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources:
<source-resource-logical-id>
: Type:<resource-type>
... Connectors:<connector-name>
: Properties: Destination:<properties-that-identify-destination-resource>
Permissions:<permission-types-to-provision>
...
Example of connectors
In this example, we use connectors to write data from an AWS Lambda function to an Amazon DynamoDB table.
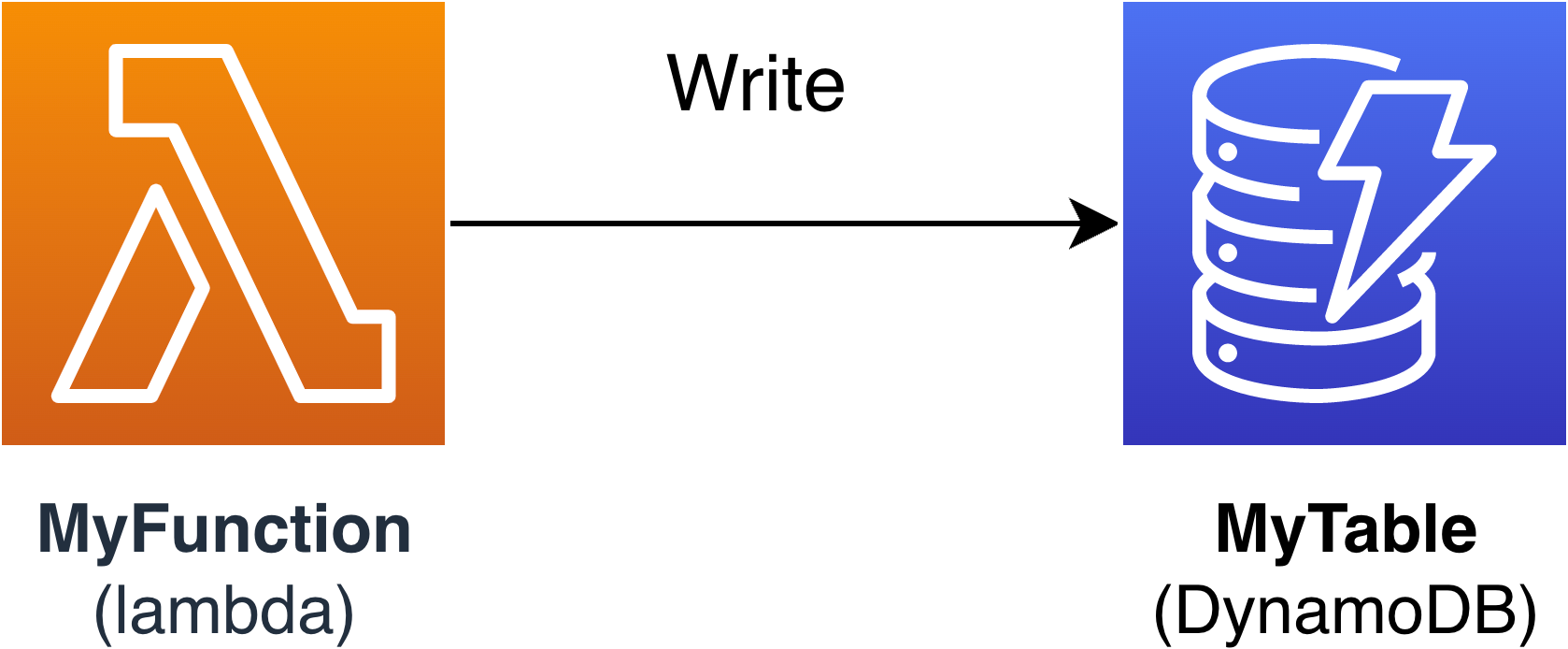
Transform: AWS::Serverless-2016-10-31 Resources: MyTable: Type: AWS::Serverless::SimpleTable MyFunction: Type: AWS::Serverless::Function Connectors: MyConn: Properties: Destination: Id: MyTable Permissions: - Write Properties: Runtime: nodejs16.x Handler: index.handler InlineCode: | const AWS = require("aws-sdk"); const docClient = new AWS.DynamoDB.DocumentClient(); exports.handler = async (event, context) => { await docClient.put({ TableName: process.env.TABLE_NAME, Item: { id: context.awsRequestId, event: JSON.stringify(event) } }).promise(); } Environment: Variables: TABLE_NAME: !Ref MyTable
The Connectors
resource attribute is embedded within the Lambda function source resource. The
DynamoDB table is defined as the destination resource using the Id
property. Connectors will provision
Write
permissions between these two resources.
When you deploy your AWS SAM template to AWS CloudFormation, AWS SAM will automatically compose the necessary access policies required for this connection to work.
Supported connections between source and destination resources
Connectors support Read
and Write
data and event permission types between a select
combination of source and destination resource connections. For example, connectors support a Write
connection between an AWS::ApiGateway::RestApi
source resource and an AWS::Lambda::Function
destination resource.
Source and destination resources can be defined by using a combination of supported properties. Property requirements will depend on the connection you are making and where the resources are defined.
Note
Connectors can provision permissions between supported serverless and non-serverless resource types.
For a list of supported resource connections and their property requirements, see Supported source and destination resource types for connectors.
Using connectors
Define Read and Write permissions
Read
and Write
permissions can be provisioned within a single connector:
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources: MyFunction: Type: AWS::Lambda::Function Connectors: MyTableConn: Properties: Destination: Id: MyTable Permissions: - Read - Write MyTable: Type: AWS::DynamoDB::Table
Define resources by using other supported properties
For both source and destination resources, when defined within the same template, use the Id
property. Optionally, a Qualifier
can be added to narrow the scope of your defined resource. When
the resource is not within the same template, use a combination of supported properties.
-
For a list of supported property combinations for source and destination resources, see Supported source and destination resource types for connectors.
-
For a description of properties that you can use with connectors, see AWS::Serverless::Connector.
When you define a source resource with a property other than Id
, use the
SourceReference
property.
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources:
<source-resource-logical-id>
: Type:<resource-type>
... Connectors:<connector-name>
: Properties: SourceReference: Qualifier:<optional-qualifier>
<other-supported-properties>
Destination:<properties-that-identify-destination-resource>
Permissions:<permission-types-to-provision>
Here's an example, using a Qualifier
to narrow the scope of an Amazon API Gateway resource:
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources: MyApi: Type: AWS::Serverless::Api Connectors: ApiToLambdaConn: Properties: SourceReference: Qualifier: Prod/GET/foobar Destination: Id: MyFunction Permissions: - Write ...
Here's an example, using a supported combination of Arn
and Type
to define a
destination resource from another template:
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources: MyFunction: Type: AWS::Serverless::Function Connectors: TableConn: Properties: Destination: Type: AWS::DynamoDB::Table Arn: !GetAtt MyTable.Arn ...
Create multiple connectors from a single source
Within a source resource, you can define multiple connectors, each with a different destination resource.
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources: MyFunction: Type: AWS::Serverless::Function Connectors: BucketConn: Properties: Destination: Id: MyBucket Permissions: - Read - Write SQSConn: Properties: Destination: Id: MyQueue Permissions: - Read - Write TableConn: Properties: Destination: Id: MyTable Permissions: - Read - Write TableConnWithTableArn: Properties: Destination: Type: AWS::DynamoDB::Table Arn: !GetAtt MyTable.Arn Permissions: - Read - Write ...
Create multi-destination connectors
Within a source resource, you can define a single connector with multiple destination resources. Here's an example of a Lambda function source resource connecting to an Amazon Simple Storage Service (Amazon S3) bucket and DynamoDB table:
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources: MyFunction: Type: AWS::Serverless::Function Connectors: WriteAccessConn: Properties: Destination: - Id: OutputBucket - Id: CredentialTable Permissions: - Write ... OutputBucket: Type: AWS::S3::Bucket CredentialTable: Type: AWS::DynamoDB::Table
Define resource attributes with connectors
Resource attributes can be defined for resources to specify additional behaviors and relationships. To learn more about resource attributes, see Resource attribute reference in the AWS CloudFormation User Guide.
You can add resource attributes to your embedded connector by defining them on the same level as your connector properties. When your AWS SAM template is transformed at deployment, attributes will pass through to the generated resources.
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources: MyFunction: Type: AWS::Serverless::Function Connectors: MyConn: DeletionPolicy: Retain DependsOn: AnotherFunction Properties: ...
How connectors work
Note
This section explains how connectors provision the necessary resources behind the scenes. This happens for you automatically when using connectors.
First, the embedded Connectors
resource attribute is transformed into an
AWS::Serverless::Connector
resource type. Its logical ID is automatically created as
<source-resource-logical-id><embedded-connector-logical-id>
.
For example, here is an embedded connector:
AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 ... Resources: MyFunction: Type: AWS::Lambda::Function Connectors: MyConn: Properties: Destination: Id: MyTable Permissions: - Read - Write MyTable: Type: AWS::DynamoDB::Table
This will generate the following AWS::Serverless::Connector
resource:
Transform: AWS::Serverless-2016-10-31 Resources: ... MyFunctionMyConn: Type: AWS::Serverless::Connector Properties: Source: Id: MyFunction Destination: Id: MyTable Permissions: - Read - Write
Note
You can also define connectors in your AWS SAM template by using this syntax. This is recommended when your source resource is defined on a separate template from your connector.
Next, the necessary access policies for this connection are automatically composed. For more information about the resources generated by connectors, see AWS CloudFormation resources generated when you specify AWS::Serverless::Connector.
Benefits of AWS SAM connectors
By automatically composing the appropriate access policies between resources, connectors provide you the ability to author your serverless applications and focus on your application architecture without needing expertise in AWS authorization capabilities, policy language, and service-specific security settings. Therefore, connectors are a great benefit to developers who may be new to serverless development, or seasoned developers looking to increase their development velocity.
Learn more
For more information about using AWS SAM connectors, see AWS::Serverless::Connector.
Provide feedback
To provide feedback on connectors, submit a new issue