Integrate Amazon Connect chat into a mobile application
The topics in this section explain how to build a custom Amazon Connect chat UI in your mobile application. This requires using your own chat back end. You must also use the Amazon Connect StartChatContact API to initiate contact, and the Participant Service APIs for managing chat participation.
Note
The StartChatContact
API requires AWS Signature Version 4 signing.
Therefore, initial requests should be routed through your personal chat back end. Subsequent API calls to the
Amazon Connect Participant Service (ACPS) can be handled directly from the mobile application.
Topics
Integration workflow
The following diagram shows the programming flow between a customer using a mobile app and an agent. Numbered text in the diagram corresponds to numbered text below the image.
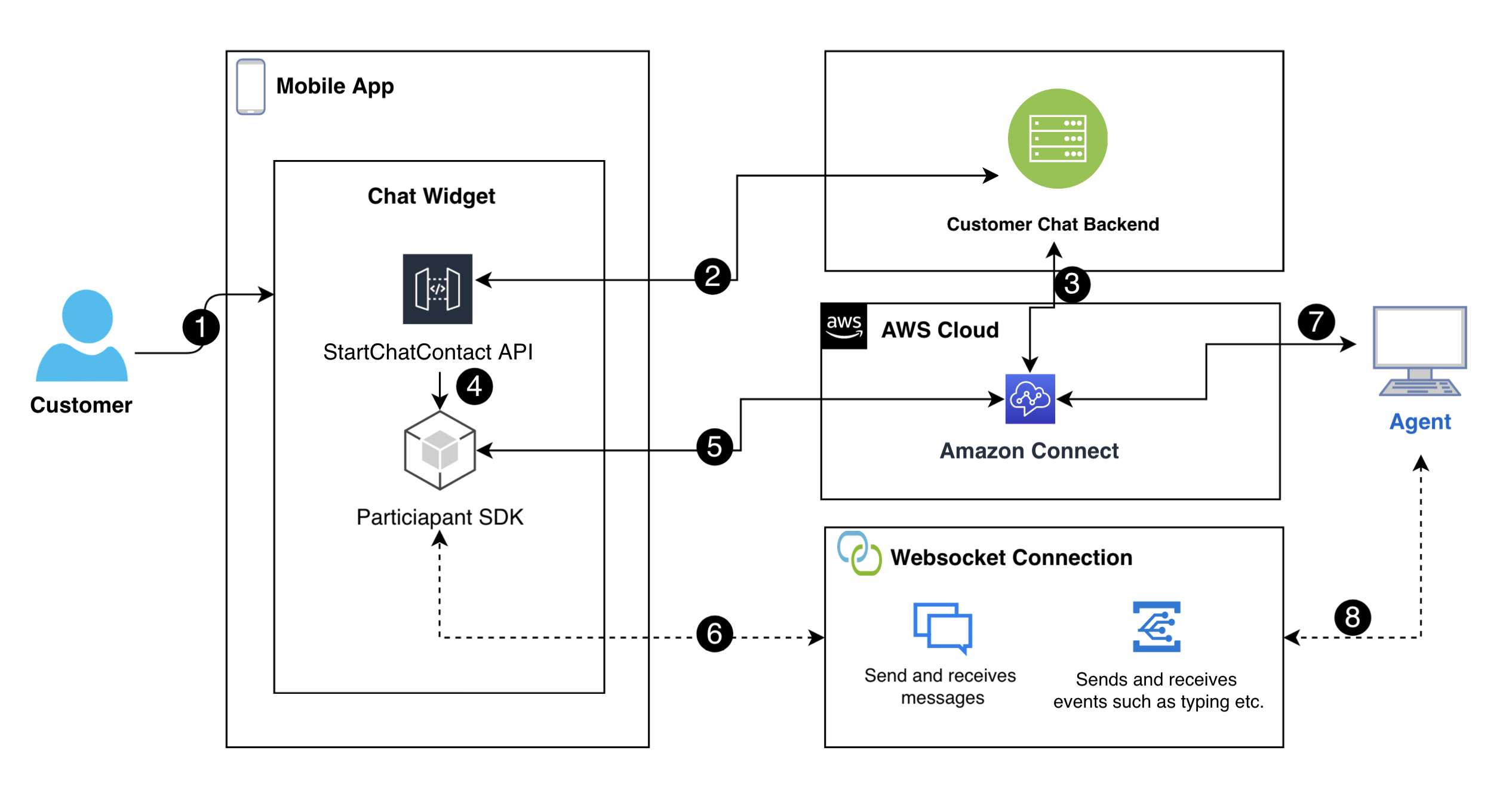
In the diagram:
When a customer starts a chat in the mobile app, the app sends a request to Amazon Connect using the StartChatContact API. This requires specific parameters, such as the API endpoint and IDs for the instance and contact flow to authenticate and initiate the chat.
The
StartChatContact
API interacts with your back-end system to obtain a participant token and a contact ID that act as unique identifiers for the chat session.The app's back end uses the participant token and contact ID to communicate with Amazon Connect, setting up the customer's chat session.
The Amazon Connect Participant SDK is activated using the participant token, preparing the app for the chat.
The Participant SDK uses the session details to establish a secure line of communication with Amazon Connect.
Amazon Connect responds by providing a WebSocket URL through the Participant SDK. The app uses this URL to create a WebSocket connection for real-time messaging.
Amazon Connect assigns a support agent to the chat based on the contact flow and routing profile.
Messages from the agent come through the WebSocket connection. The mobile app listens to the WebSocket to receive and display messages from the agent, and to send customer messages back.
Prerequisites
You must have the following prerequisites in order to integrate Amazon Connect chat with a mobile app:
-
Create an Amazon Connect instance.
βorβ
If you have a Connect instance, follow these steps on GitHub to enable chat for the instance
. -
Create an Amazon Connect Contact Flow, ready to receive chat contacts.
Note the instance ID.
Find the contactFlowId for the Sample inbound flow (first contact experience).
-
Deploy a custom Amazon Connect chat back end. Refer to the startChatContactAPI template
on GitHub. Deploy a startChatContact
Lambda function CloudFront template. Add the
region
,API_GATEWAY_ID
,contactFlowId
, andinstanceID
to the template's Config file.
Install libraries
You start the integration process by installing the libraries for iOS and Android. You must install both sets of libraries.
-
iOS: Go to the aws-sdk-ios
repository on GitHub and install the following: pod 'AWSCore' pod 'AWSConnectParticipant' pod 'Starscream', '~> 4.0'
-
Android: Go to the aws-sdk-android
repository on GitHub and install the following: implementation("com.amazonaws:aws-android-sdk-core:2.73.0") implementation("com.amazonaws:aws-android-sdk-connectparticipant:2.73.0")
Configure AWS credentials
After you install both sets of libraries, you register with the Amazon Connect Service and the
Amazon Connect Participant Service. To do that, in the Config files for each operating system,
use your AWS credentials and replace the accessKey
and
secretKey
values with empty strings (ββ
) as shown in the
following examples.
Important
You must specify the Region you are testing in.
-
iOS: Use
AWSStaticCredentialsProvider
for Amazon Connect Service and Participant Service registration.private let config = Config() let credentials = AWSStaticCredentialsProvider(accessKey: "", secretKey: "") let participantService = AWSServiceConfiguration(region: config.region, credentialsProvider: credentials)! AWSConnectParticipant.register(with: participantService, forKey: "") connectParticipantClient = AWSConnectParticipant.init(forKey: "")
-
Android: Initialize
AmazonConnectParticipantAsyncClient
and set the Region.private var connectParticipantClient: AmazonConnectParticipantAsyncClient = AmazonConnectParticipantAsyncClient() private val chatConfiguration = Config init { connectParticipantClient.setRegion(Region.getRegion(chatConfiguration.
region
)) }
Note
You can download the Config files from GitHub. You must edit both Config files.
Start a chat
Next, you must integrate the StartChatContact API provided by Amazon Connect. The API starts the conversation by registering the customer's intent to chat with an agent.
Note
To deploy a StartChatContact
Lambda function, use the
CloudFront template
For iOS and Android, the StartChatContact
API call requires several pieces of information:
InstanceId
: The identifier of the Amazon Connect instance.ContactFlowId
: The identifier of the contact flow to handle the chat.ParticipantDetails
: Information about the participant, such as the customer's display name.Attributes
: Additional information about the contact that may be useful for routing or handling the chat within Amazon Connect.
Important
You must use AWS Signature Version 4 (SigV4) to sign the API call. The SigV4 process adds authentication information to AWS API requests sent via HTTP. For mobile clients, we recommend performing the signing process on the server side. Your mobile app sends a request to your server, which then signs the request and forwards it to Amazon Connect. This helps secure your AWS credentials.
For iOS:
func startChatSession(displayName: String, completion: @escaping (Result<StartChatResponse, Error>) -> Void) { // Your back end server will handle SigV4 signing and make the API call to Amazon Connect // Use the completion handler to process the response or error }
For Android:
// Make a network call to your back end server suspend fun startChatSession(displayName: String): StartChatResponse { // Your back end server will handle SigV4 signing if needed and make the // API call to Amazon Connect // Handle the response or error accordingly }
Create a participant connection
You use the details received from a StartChatContact API call to create a participant connection. You then call the AWS Connect participant SDK, which return the WebSocket URL needed to establish the connection.
The following example shows how to establish a connection for iOS:
// Swift code snippet for iOS participant connection setup /// Creates the participant's connection. https://docs.aws.amazon.com/connect-participant/latest/APIReference/API_CreateParticipantConnection.html /// - Parameter: participantToken: The ParticipantToken as obtained from StartChatContact API response. func createParticipantConnection() { let createParticipantConnectionRequest = AWSConnectParticipantCreateParticipantConnectionRequest() createParticipantConnectionRequest?.participantToken = self.participantToken createParticipantConnectionRequest?.types = ["WEBSOCKET", "CONNECTION_CREDENTIALS"] connectParticipantClient? .createParticipantConnection (createParticipantConnectionRequest!) .continueWith(block: { (task) -> Any? in self.connectionToken = task.result!.connectionCredentials!.connectionToken self.websocketUrl = task.result!.websocket!.url return nil } ).waitUntilFinished() }
The following example shows how to establish a connection for Android:
// Kotlin code snippet for Android participant connection setup /// Creates the participant's connection. https://docs.aws.amazon.com/connect-participant/latest/APIReference/API_CreateParticipantConnection.html /// - Parameter: participantToken: The ParticipantToken as obtained from StartChatContact API response. fun createParticipantConnection( _participantToken: String, handler: AsyncHandler<CreateParticipantConnectionRequest, CreateParticipantConnectionResult> ) { val createParticipantConnectionRequest = CreateParticipantConnectionRequest().apply { setType(listOf("WEBSOCKET", "CONNECTION_CREDENTIALS")) participantToken = _participantToken } connectParticipantClient.createParticipantConnectionAsync( createParticipantConnectionRequest, handler ) }
Use WebSocket connections
Use the Participant SDK to obtain a WebSocket URL for the chat connection.
To implement WebSocket management, use existing solutions or implement your own.
To handle Websocket messages and events, implement your own solution, or use our solutions for iOS
and for Android . -
Ensure that you cover all message and event types:
case
typing = "application/vnd.amazonaws.connect.event.typing"
case
messageDelivered = "application/vnd.amazonaws.connect.event.message.delivered"
case
messageRead = "application/vnd.amazonaws.connect.event.message.read"
case
metaData = "application/vnd.amazonaws.connect.event.message.metadata"
case
joined = "application/vnd.amazonaws.connect.event.participant.joined"
case
left = "application/vnd.amazonaws.connect.event.participant.left"
case
ended = "application/vnd.amazonaws.connect.event.chat.ended"
case
plainText = "text/plain"
case
richText = "text/markdown"
case
interactiveText = "application/vnd.amazonaws.connect.message.interactive"
The following image shows a default instance of Connect chat. Numbers in the image correspond to numbered text below.
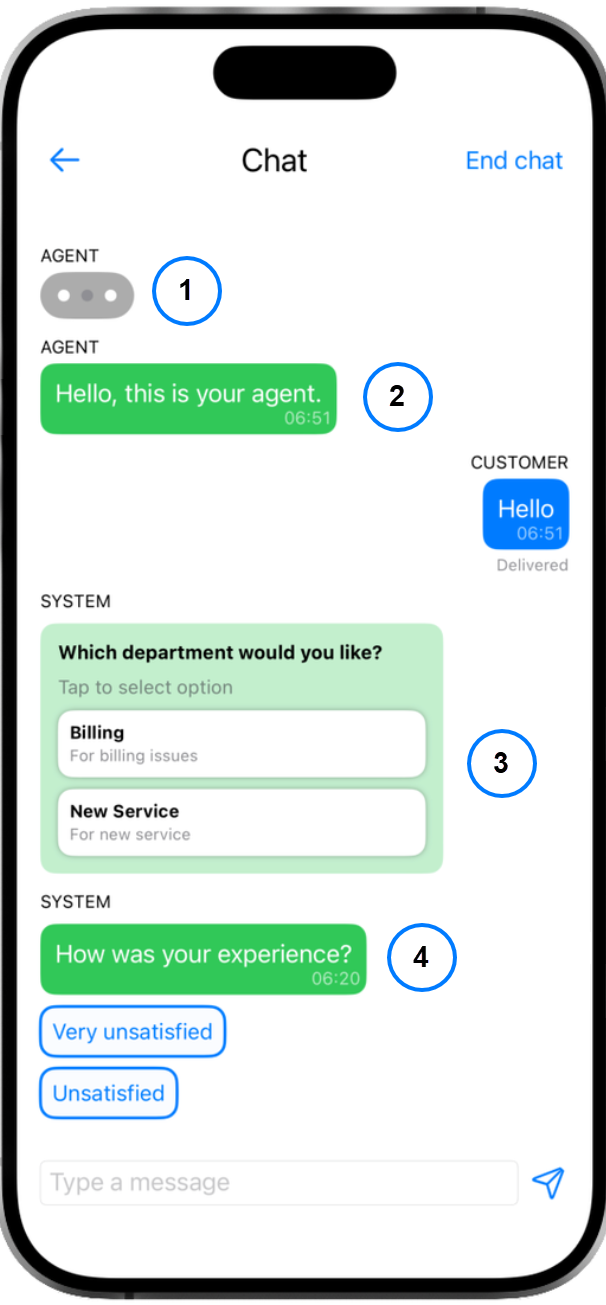
In the image, starting near the top:
The typing indicator shows who is typing a message.
This example uses plain-text messages. You can enable the use of markdown if desired.
The interactive list control provides users with lists of choices, such as customer service or billing.
Interactive quick replies provide pre-programmed responses to common questions or phrases.
Send messages
You use the SendMessage
function to send chat messages.
/// To send a message using the Participant SDK. /// - Parameters: /// - messageContent: The content of the message. /// - connectionToken: The authentication token associated with the connection - Received from Participant Connection /// - contentType: text/plain, text/markdown, application/json, and application/vnd.amazonaws.connect.message.interactive.response func sendChatMessage(messageContent: String) { let sendMessageRequest = AWSConnectParticipantSendMessageRequest() sendMessageRequest?.connectionToken = self.connectionToken sendMessageRequest?.content = messageContent sendMessageRequest?.contentType = "text/plain" connectParticipantClient? .sendMessage(sendMessageRequest!) .continueWith(block: { (task) -> Any? in return nil }) }
Handle events
Use the SendEvent
function in the Participant SDK for events such as
typing and read receipts.
/// Sends an event such as typing, joined, left etc. /// - Parameters: /// - contentType: The content type of the request /// - content: The content of the event to be sent (for example, message text). For content related to message receipts, this is supported in the form of a JSON string. func sendEvent(contentType: ContentType, content: String = "") { let sendEventRequest = AWSConnectParticipantSendEventRequest() sendEventRequest?.connectionToken = self.connectionToken sendEventRequest?.contentType = contentType.rawValue sendEventRequest?.content = content // Set the content here connectParticipantClient? .sendEvent(sendEventRequest!) .continueWith(block: { (task) -> Any? in return nil }) }
End a chat
To end a chat, use the disconnectParticipant
function in the Participant
SDK.
/// Disconnects a participant. /// - Parameter: connectionToken: The authentication token associated with the connection - Received from Participant Connection func endChat() { let disconnectParticipantRequest = AWSConnectParticipantDisconnectParticipantRequest() disconnectParticipantRequest?.connectionToken = self.connectionToken connectParticipantClient?.disconnectParticipant(disconnectParticipantRequest!) .continueWith(block: { (task) -> Any? in return nil }).waitUntilFinished() self.websocketUrl = nil }
Source code
The following sample projects on GitHub show how to implement Connect chat.
iOS: iOS Native Chat Demo
. Android: Android Native Chat Demo
.