AWS SDK for JavaScript V3 APIリファレンスガイドでは、バージョン 3 (V3) のすべてのAPIオペレーション AWS SDK for JavaScript について詳しく説明します。
翻訳は機械翻訳により提供されています。提供された翻訳内容と英語版の間で齟齬、不一致または矛盾がある場合、英語版が優先します。
DynamoDB にデータを送信するアプリケーションを構築します
このクロスサービス Node.js チュートリアルでは、ユーザーが Amazon DynamoDB 表にデータの送信を有効にするアプリケーションを構築する方法について示します。このアプリケーションでは、次のサービスを使用します。
AWS Identity and Access Management(IAM) と Amazon Cognito の認可と許可。
表を作成および更新するための「Amazon DynamoDB」(Amazon DynamoDB)。
Amazon Simple Notification Service (Amazon SNS) は、ユーザーが表を更新したときにアプリケーション管理者に通知します。
シナリオ
このチュートリアルでは、HTML ページが Amazon DynamoDB表にデータを送信するためのブラウザベースのアプリケーションを提供します。アプリケーションは Amazon SNS を使用して、ユーザーが表を更新したときにアプリケーション管理者に通知します。
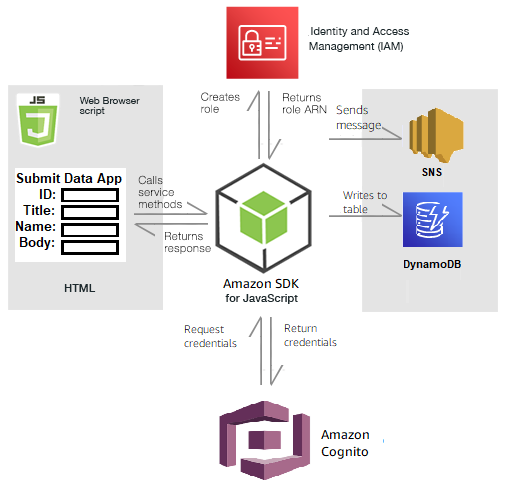
アプリを構築するには
前提条件
以下の前提条件を満たしてください。
-
これらの Node TypeScriptの例を実行するようにプロジェクト環境を設定し、必要なAWS SDK for JavaScriptとサードパーティーのモジュールをインストールします。「GitHub
」の指示に従います。 ユーザーの認証情報を使用して、共有設定ファイルを作成します。共有認証情報ファイルの提供の詳細については、「AWS SDK とツールのリファレンスガイド」の「共有設定ファイルおよび認証情報ファイル」を参照してください。
AWS リソースを作成する
このアプリケーションは、次のリソースが必要になります。
次の権限を持つ AWS Identity and Access Management (IAM) 認証されていない Amazon Cognito ユーザーロール
sns:Publish
DynamoDB: PutItem
DynamoDB 表。
これらのリソースは、AWS コンソールで手動で作成することができますが、このチュートリアルで説明するように AWS CloudFormation を使用してこれらのリソースをプロビジョニングすることをお勧めします。
AWS CloudFormation を使用して AWS リソースを作成する
AWS CloudFormationは、AWSインフラストラクチャデプロイを予想可能および繰り返し作成し、プロビジョニングすることができます。AWS CloudFormation についてはAWS CloudFormationユーザーガイドを参照してください。
AWS CLI を使用して AWS CloudFormation スタックを作成するには:
「AWS CLI ユーザーガイド」の手順に従って AWS CLI をインストールして設定します。
プロジェクトフォルダのルートディレクトリで、
setup.yaml
という名前のファイルを作成し、それにこの GitHubにコンテンツをコピーします。 注記
AWS CloudFormation テンプレートは、この GitHub
で公開されている AWS CDK を使用して生成されました。AWS CDK の詳細については、AWS Cloud Development Kit (AWS CDK) デベロッパーガイドを参照してください。 コマンドラインから以下のコマンドを実行し、
STACK_NAME
をスタックの一意の名前に置き換え、REGION
を AWS リージョンに置き換えます。重要
スタック名は、AWS 地域および AWS アカウント内で一意である必要があります。最大 128 文字まで指定でき、数字とハイフンを使用できます。
aws cloudformation create-stack --stack-name STACK_NAME --template-body file://setup.yaml --capabilities CAPABILITY_IAM --region REGION
create-stack
コマンドパラメータの詳細については、 AWS CLI Command Reference guide(コマンドリファレンスガイド)およびAWS CloudFormation User Guide(ユーザーガイド)を参照してください。作成されたリソースを表示するには、AWSマネジメントコンソールでAWS CloudFormationを開き、スタックを選択し、Resources(リソース)タブを選択します。
スタックが作成されたら、表に入力しますの説明のようにAWS SDK for JavaScriptを使用して DynamoDB表に入力します。
表に入力します
テーブルにデータを入力するには、まず libs
という名前のディレクトリを作成し、そこに dynamoClient.js
という名前のファイルを作成し、それに以下の内容を貼り付けます。REGION
を実際の AWS リージョンに置き換え、IDENTITY_POOL_ID
を Amazon Cognito アイデンティティプール ID に置き換えます。これにより DynamoDB クライアントオブジェクトが作成されます。
import { CognitoIdentityClient } from "@aws-sdk/client-cognito-identity"; import { fromCognitoIdentityPool } from "@aws-sdk/credential-provider-cognito-identity"; import { DynamoDBClient } from "@aws-sdk/client-dynamodb"; const REGION = "REGION"; const IDENTITY_POOL_ID = "IDENTITY_POOL_ID"; // An Amazon Cognito Identity Pool ID. // Create an Amazon DynaomDB service client object. const dynamoClient = new DynamoDBClient({ region: REGION, credentials: fromCognitoIdentityPool({ client: new CognitoIdentityClient({ region: REGION }), identityPoolId: IDENTITY_POOL_ID, }), }); export { dynamoClient };
このコードはこのGitHubに
次に、プロジェクトフォルダのdynamoAppHelperFiles
フォルダを作成、そこにファイルupdate-table.js
を作成し、このGitHub
// Import required AWS SDK clients and commands for Node.js import { PutItemCommand } from "@aws-sdk/client-dynamodb"; import { dynamoClient } from "../libs/dynamoClient.js"; // Set the parameters export const params = { TableName: "Items", Item: { id: { N: "1" }, title: { S: "aTitle" }, name: { S: "aName" }, body: { S: "aBody" }, }, }; export const run = async () => { try { const data = await dynamoClient.send(new PutItemCommand(params)); console.log("success"); console.log(data); } catch (err) { console.error(err); } }; run();
コマンドラインから、以下のコマンドを実行します。
node update-table.js
このコードはこのGitHubに
アプリケーションのフロントエンドページを作成します
ここでは、アプリケーションのフロントエンド HTMLブラウザページを作成します。
DynamoDBApp
ディレクトリを作成し、index.html
という名前のファイルを作成し、GitHub のここscript
要素は、例に必要なすべてのJavaScriptを含むmain.js
ファイルを追加します。このチュートリアルの後半で、main.js
ファイルを作成します。index.html
の残りのコードは、ユーザーが入力するデータをキャプチャするブラウザページを作成します。
このサンプルコードは、この GitHub に
ブラウザスクリプトを作成する
まず、この例に必要なサービスクライアントオブジェクトを作成します。libs
ディレクトリの作成、snsClient.js
を作成し、それに以下のコードをペーストします。それぞれのREGION
(地域)とIDENTITY_POOL_ID
(アイデンティティプールID)を置き換えます。
注記
AWS リソースを作成する で作成したAmazon CognitoアイデンティティプールのIDを使用します。
import { CognitoIdentityClient } from "@aws-sdk/client-cognito-identity"; import { fromCognitoIdentityPool } from "@aws-sdk/credential-provider-cognito-identity"; import { SNSClient } from "@aws-sdk/client-sns"; const REGION = "REGION"; const IDENTITY_POOL_ID = "IDENTITY_POOL_ID"; // An Amazon Cognito Identity Pool ID. // Create an Amazon Comprehend service client object. const snsClient = new SNSClient({ region: REGION, credentials: fromCognitoIdentityPool({ client: new CognitoIdentityClient({ region: REGION }), identityPoolId: IDENTITY_POOL_ID, }), }); export { snsClient };
このコードは このGitHubに
この例のブラウザースクリプトを作成するには、DynamoDBApp
というフォルダに、ファイル名add_data.js
で Node.js モジュールを作成し、それに以下のコードをペーストします。submitData
関数は DynamoDB 表にデータを送信し、Amazon SNSを使用してアプリケーション管理者にSMSテキストを送信します。
submitData
関数で、ターゲットの電話番号、アプリケーションインターフェイスで入力された値、および Amazon S3 バケットの名前の変数を表します。次に、表に項目を追加するためのパラメータオブジェクトを作成します。いずれの値も空でない場合は、submitData
が表に項目を追加し、メッセージが送信されます。関数を window.submitData = submitData
でブラウザに利用可能にすることを忘れないでください。
// Import required AWS SDK clients and commands for Node.js import { PutItemCommand } from "@aws-sdk/client-dynamodb"; import { PublishCommand } from "@aws-sdk/client-sns"; import { snsClient } from "../libs/snsClient.js"; import { dynamoClient } from "../libs/dynamoClient.js"; export const submitData = async () => { //Set the parameters // Capture the values entered in each field in the browser (by id). const id = document.getElementById("id").value; const title = document.getElementById("title").value; const name = document.getElementById("name").value; const body = document.getElementById("body").value; //Set the table name. const tableName = "Items"; //Set the parameters for the table const params = { TableName: tableName, // Define the attributes and values of the item to be added. Adding ' + "" ' converts a value to // a string. Item: { id: { N: id + "" }, title: { S: title + "" }, name: { S: name + "" }, body: { S: body + "" }, }, }; // Check that all the fields are completed. if (id != "" && title != "" && name != "" && body != "") { try { //Upload the item to the table await dynamoClient.send(new PutItemCommand(params)); alert("Data added to table."); try { // Create the message parameters object. const messageParams = { Message: "A new item with ID value was added to the DynamoDB", PhoneNumber: "PHONE_NUMBER", //PHONE_NUMBER, in the E.164 phone number structure. // For example, ak standard local formatted number, such as (415) 555-2671, is +14155552671 in E.164 // format, where '1' in the country code. }; // Send the SNS message const data = await snsClient.send(new PublishCommand(messageParams)); console.log( "Success, message published. MessageID is " + data.MessageId, ); } catch (err) { // Display error message if error is not sent console.error(err, err.stack); } } catch (err) { // Display error message if item is no added to table console.error( "An error occurred. Check the console for further information", err, ); } // Display alert if all field are not completed. } else { alert("Enter data in each field."); } }; // Expose the function to the browser window.submitData = submitData;
このサンプルコードは、このGitHubに
最後に、コマンドプロンプトで以下を実行して、この例の JavaScript をmain.js
という名前のファイルにバンドルします。
webpack add_data.js --mode development --target web --devtool false -o main.js
注記
webpackのインストールについては、「Webpack でアプリケーションをバンドルする」を参照してください。
アプリケーションを実行するには、ブラウザでindex.html
を開きます。
リソースを削除します
このチュートリアルの冒頭で説明したように、このチュートリアルを進めるうえで作成したすべてのリソースを終了して料金が発生しないようにしてください。これを行うには、このチュートリアルの AWS リソースを作成する トピックで作成した AWS CloudFormation スタックを以下のように削除します。
「スタック」ページを開き、スタックを選択します。
削除 を選択します。
AWS クロスサービスの例の詳細については、「AWS SDK for JavaScript クロスサービスの例」を参照してください。