Set up the AWS SDK for Swift
The AWS SDK for Swift is a cross-platform, open source Swift
The SDK package is imported into your project using the Swift Package Manager
Topics
Prerelease documentation
This is prerelease documentation for an SDK in preview release. It may be incomplete and is subject to change.
In addition, versions of the SDK prior to version 1.0.0 may have flaws, and no guarantee is made about the API’s stability. Changes can and will occur that break compatibility during the prerelease stage. These releases are not intended for use in production code!
When you’ve finished following the steps in this article, or have confirmed that everything is configured as described, you’re ready to begin developing using the AWS SDK for Swift.
Overview
To make requests to AWS using the AWS SDK for Swift, you need the following:
-
An active AWS account.
-
A user in IAM Identity Center with permission to use the AWS services and resources your application will access.
-
A development environment with version 5.7 or later of the Swift toolchain. If you don’t have this, you’ll install it as part of the steps below.
-
Xcode users need version 14 or later of the Xcode application.
After finishing these steps, you’re ready to use the SDK to develop Swift projects that access AWS services.
Set up AWS access
Before installing the Swift tools, configure your environment to let your project access AWS services. This section covers creating and configuring your AWS account, preparing IAM Identity Center for use, and setting the environment variables used by the SDK for Swift to fetch your access credentials.
Sign up for an AWS account
If you do not have an AWS account, complete the following steps to create one.
To sign up for an AWS account
Follow the online instructions.
Part of the sign-up procedure involves receiving a phone call and entering a verification code on the phone keypad.
When you sign up for an AWS account, an AWS account root user is created. The root user has access to all AWS services and resources in the account. As a security best practice, assign administrative access to a user, and use only the root user to perform tasks that require root user access.
AWS sends you a confirmation email after the sign-up process is
complete. At any time, you can view your current account activity and manage your account by
going to https://aws.amazon.com/
Create a user with administrative access
After you sign up for an AWS account, secure your AWS account root user, enable AWS IAM Identity Center, and create an administrative user so that you don't use the root user for everyday tasks.
Secure your AWS account root user
-
Sign in to the AWS Management Console
as the account owner by choosing Root user and entering your AWS account email address. On the next page, enter your password. For help signing in by using root user, see Signing in as the root user in the AWS Sign-In User Guide.
-
Turn on multi-factor authentication (MFA) for your root user.
For instructions, see Enable a virtual MFA device for your AWS account root user (console) in the IAM User Guide.
Create a user with administrative access
-
Enable IAM Identity Center.
For instructions, see Enabling AWS IAM Identity Center in the AWS IAM Identity Center User Guide.
-
In IAM Identity Center, grant administrative access to a user.
For a tutorial about using the IAM Identity Center directory as your identity source, see Configure user access with the default IAM Identity Center directory in the AWS IAM Identity Center User Guide.
Sign in as the user with administrative access
-
To sign in with your IAM Identity Center user, use the sign-in URL that was sent to your email address when you created the IAM Identity Center user.
For help signing in using an IAM Identity Center user, see Signing in to the AWS access portal in the AWS Sign-In User Guide.
Assign access to additional users
-
In IAM Identity Center, create a permission set that follows the best practice of applying least-privilege permissions.
For instructions, see Create a permission set in the AWS IAM Identity Center User Guide.
-
Assign users to a group, and then assign single sign-on access to the group.
For instructions, see Add groups in the AWS IAM Identity Center User Guide.
Grant programmatic AWS account access
Users need programmatic access if they want to interact with AWS outside of the AWS Management Console. The way to grant programmatic access depends on the type of user that's accessing AWS.
To grant users programmatic access, choose one of the following options.
Which user needs programmatic access? | To | By |
---|---|---|
Workforce identity (Users managed in IAM Identity Center) |
Use temporary credentials to sign programmatic requests to the AWS CLI, AWS SDKs, or AWS APIs. |
Following the instructions for the interface that you want to use.
|
IAM | Use temporary credentials to sign programmatic requests to the AWS CLI, AWS SDKs, or AWS APIs. | Following the instructions in Using temporary credentials with AWS resources in the IAM User Guide. |
IAM | (Not recommended) Use long-term credentials to sign programmatic requests to the AWS CLI, AWS SDKs, or AWS APIs. |
Following the instructions for the interface that you want to use.
|
For more advanced cases regarding configuring the credentials and Region, see The .aws/credentials and .aws/config files, AWS Region, and Using environment variables in the AWS SDKs and Tools Reference Guide.
Note
If you plan to develop a macOS desktop application, keep in
mind that due to sandbox restrictions, the SDK is unable to
access your ~/.aws/config
and
~/.aws/credentials
files, as there is no
entitlement available to grant access to the
~/.aws
directory. See Security and authentication when testing
on macOS for details.
Set up your Swift development environment
The SDK for Swift requires at least version 5.7 of Swift. This can be installed either standalone or as part of the Xcode development environment on macOS.
-
Swift 5.7 toolchain or later.
-
If you're developing on macOS using Xcode, you need a minimum of Xcode 14.
-
An AWS account. If you don’t have one already, you can create one using the AWS portal
.
Prepare to install Swift
The Swift install process for Linux doesn't automatically install
libcrypto
version 1.1, even though it's required by the
compiler. To install it, be sure to install OpenSSL 1.1 or later, as
well as its development package. Using the yum
package
manager, for example:
$ sudo yum install openssl openssl-devel
Using the apt
package manager:
$ sudo apt install openssl libssl-dev
This isn't necessary on macOS.
Install Swift
On macOS, the easiest way to install Swift is to simply install
Apple’s Xcode IDE, which includes Swift and all the standard
libraries and tools that go with it. This can be found on the macOS
App Store
If you’re using Linux or Windows, or don’t want to install Xcode
on macOS, the Swift organization’s web site has detailed
instructions to install
and set up the Swift toolchain
Check the Swift tools version number
If Swift is already installed, you can verify the version number
using the command swift --version
. The output will look
similar to one of the following examples.
Checking the Swift version on macOS
$ swift --version swift-driver version: 1.87.1 Apple Swift version 5.9 (swiftlang-5.9.0.128.108 clang-1500.0.40.1) Target: x86_64-apple-macosx14.0
Checking the Swift version on Linux
$ swift --version Swift version 5.8.1 (swift-5.8.1-RELEASE) Target: x86_64-unknown-linux-gnu
For more information about the configuration and credentials files shared among the AWS Command Line Interface and the various AWS SDKs, see the AWS SDKs and Tools Reference Guide.
Security and authentication when testing on macOS
Configuring the App Sandbox
If your SDK for Swift project is a desktop application that you’re building in Xcode, you will need to enable the App Sandbox capability and turn on the "Outgoing Connections (Client)" entitlement so that the SDK can communicate with AWS.
First, open the macOS target’s Signing & Capabilities panel, shown below.
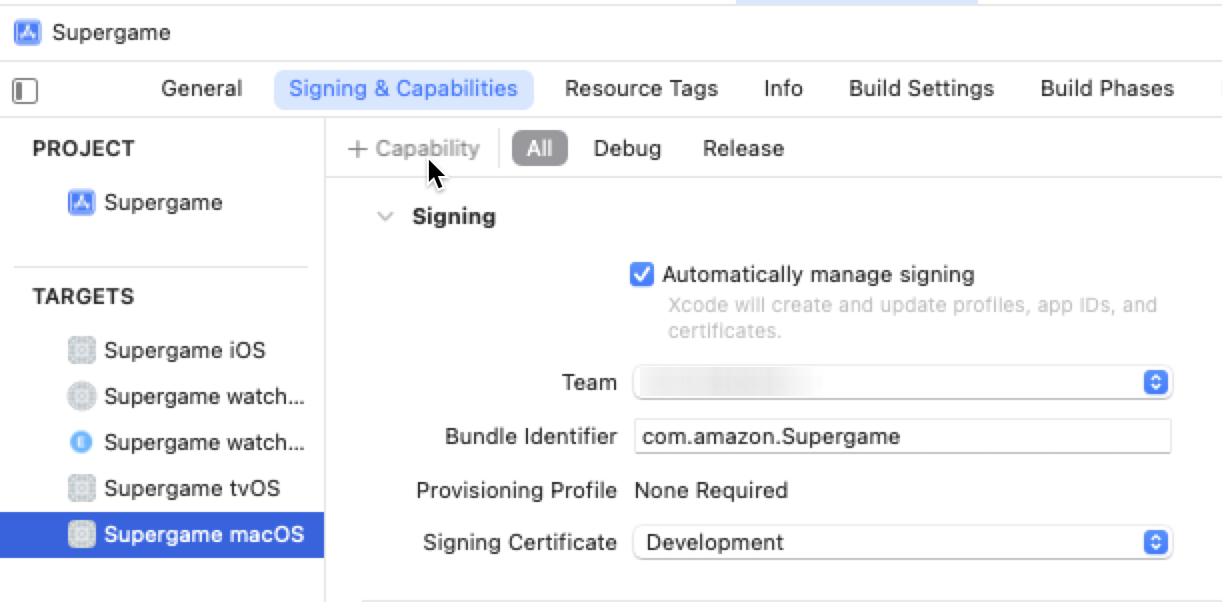
Click the + Capability button near the top left of this panel to bring up the box listing the available capabilities. In this box, locate the "App Sandbox" capability and double-click on it to add it to your target.
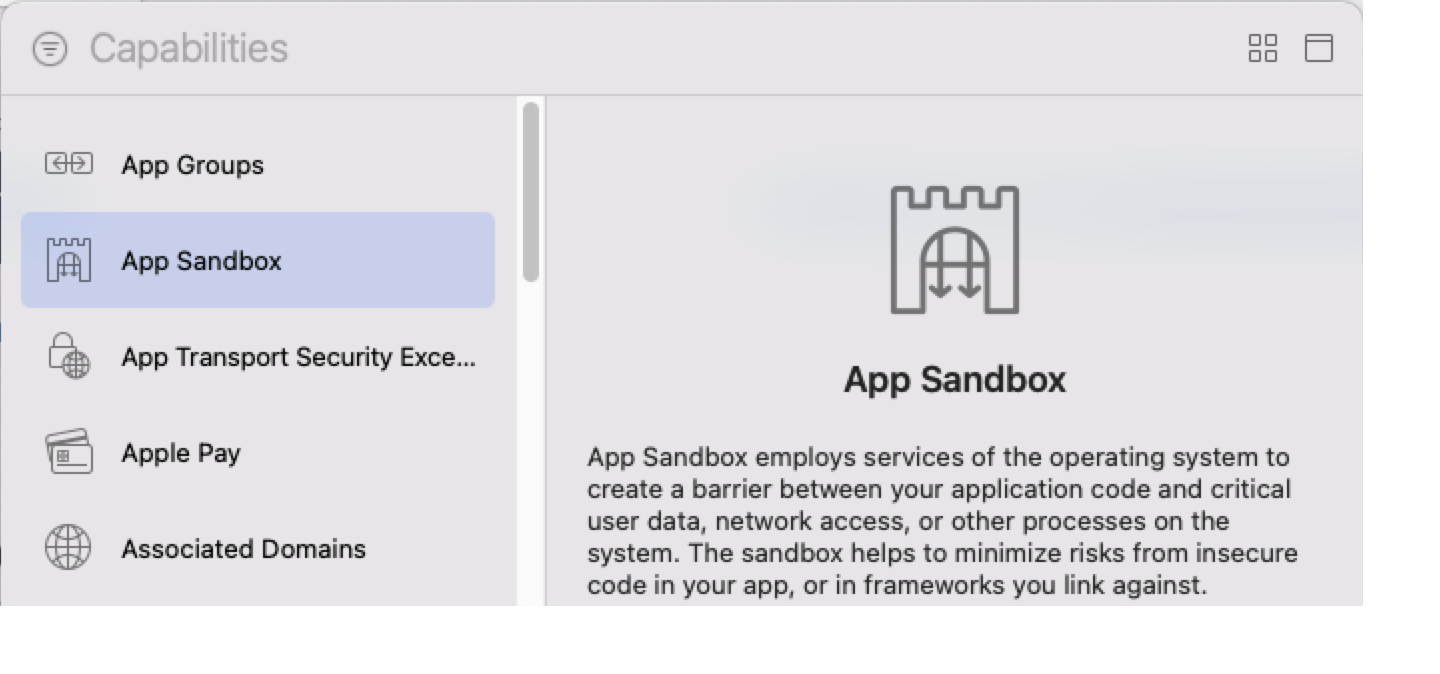
Next, back in your target’s Signing & Capabilities panel, find the new App Sandbox section and make sure that next to Network, the Outgoing Connections (Client) checkbox is selected in the following image.
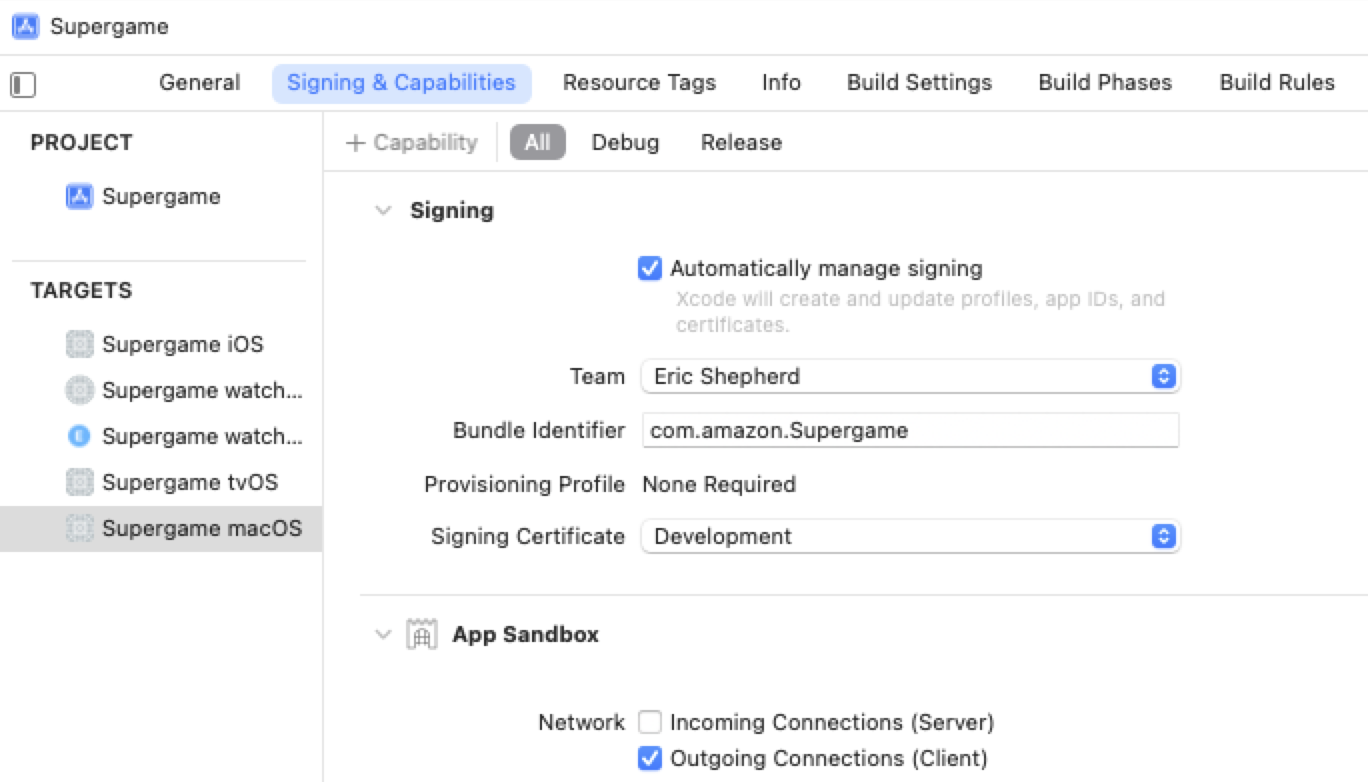
Using AWS access keys on macOS
Although shipping applications should use AWS IAM Identity Center, Amazon Cognito Identity, or similar technologies to handle authentication instead of requiring the direct use of AWS access keys, you may need to use these low-level credentials during development and testing.
macOS security features for desktop applications don’t allow
applications to access files without express user permission, so the
SDK can’t automatically configure clients using the contents of the
CLI’s ~/.aws/config
and
~/.aws/credentials
files. Instead, you need to
use the AWS_ACCESS_KEY_ID
and
AWS_SECRET_ACCESS_KEY
environment variables to specify
the authentication keys for your AWS account. This is already done
if you followed the guidance in Get started with the SDK for Swift.
If not, look there for details on setting these up.
There is a special case: when running projects from within Xcode, the environment you have set up for your shell is not automatically inherited. Because of this, if you want to test your code using your AWS account’s access key ID and secret access key, you need to set up the runtime environment for your application in the Xcode scheme for your development device. To configure the environment for a particular target in your Xcode project, switch to that target, then choose Edit Scheme in Xcode’s Product menu.
This will open the scheme editor window for your project. Click on the Run phase in the left sidebar, then Arguments in the tab bar near the top of the window.

Under Environment Variables, click the
+ icon to add AWS_REGION
and set
its value to the desired region (in the screenshot above, it’s set
to "us-east-2"). Then add AWS_ACCESS_KEY_ID
and its
value, then AWS_SECRET_ACCESS_KEY
and its value. Close
the window once you have these configured and your scheme’s Run
configuration looks similar to the above.
Important
Be sure to disable the Shared checkbox before pushing your code to any public version control repository such as GitHub. Otherwise, your AWS access key and secret access key will be included in the publicly shared content. This is important enough to be worth double-checking regularly.
Your project should now be able to use the SDK to connect to AWS services.
Next steps
Now that your tools and environment are ready for you to begin developing with AWS SDK for Swift, see Get started with the SDK for Swift, which demonstrates how to create and build a Swift project using AWS services.